java代码完成一个游戏,生成一个点击的页面,页面有16个矩形的格子。在游戏开始时,生成红色的与蓝色的8对不同的动物在格子中,红色的动物有:大象,狮子,老虎,豹子,狼,狗,猫,老鼠,蓝色的动物是:大象,狮子,老虎,豹子,狼,狗,猫,老鼠。需要显示格子中动物的颜色进行区别。动物的大小规则:大象大于狮子,狮子大于老虎,老虎大于豹子,豹子大于狼,狼大于狗,狗大于猫,猫大于老鼠,老鼠大于大象。当任意一方点击动物进行移动时可以吃掉对方的动物,需要判断当前点击的动物与目标动物大小进行比较。只有颜色不同的动物才能进行吃牌行为。当任意一方的所有的动物全部被吃时,结束游戏并提示重新开始新的游戏。
时间: 2023-07-16 11:17:19 浏览: 194
以下是Java代码的实现:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class AnimalGame extends JFrame implements ActionListener {
JButton[][] buttons;
Animal[][] animals;
AnimalColor turn;
boolean gameOver;
public AnimalGame() {
setTitle("Animal Game");
setSize(800, 800);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
buttons = new JButton[4][4];
animals = new Animal[4][4];
turn = AnimalColor.RED;
gameOver = false;
JPanel panel = new JPanel(new GridLayout(4, 4));
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
buttons[i][j] = new JButton();
buttons[i][j].setPreferredSize(new Dimension(200, 200));
buttons[i][j].addActionListener(this);
panel.add(buttons[i][j]);
}
}
getContentPane().add(panel);
setupGame();
setVisible(true);
}
public void setupGame() {
// Setup red animals
Animal[] redAnimals = {Animal.ELEPHANT, Animal.LION, Animal.TIGER, Animal.LEOPARD, Animal.WOLF, Animal.DOG, Animal.CAT, Animal.MOUSE};
shuffleArray(redAnimals);
for (int i = 0; i < 8; i++) {
animals[i / 4][i % 4] = new Animal(redAnimals[i], AnimalColor.RED);
}
// Setup blue animals
Animal[] blueAnimals = {Animal.ELEPHANT, Animal.LION, Animal.TIGER, Animal.LEOPARD, Animal.WOLF, Animal.DOG, Animal.CAT, Animal.MOUSE};
shuffleArray(blueAnimals);
for (int i = 8; i < 16; i++) {
animals[i / 4][i % 4] = new Animal(blueAnimals[i - 8], AnimalColor.BLUE);
}
updateButtons();
}
public void actionPerformed(ActionEvent e) {
if (gameOver) {
int choice = JOptionPane.showConfirmDialog(null, "Game over! Play again?", "Game over", JOptionPane.YES_NO_OPTION);
if (choice == JOptionPane.YES_OPTION) {
resetGame();
} else {
System.exit(0);
}
} else {
JButton button = (JButton)e.getSource();
int row = -1, col = -1;
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (button == buttons[i][j]) {
row = i;
col = j;
break;
}
}
}
if (animals[row][col] == null || animals[row][col].getColor() != turn) {
return;
}
boolean validMove = false;
if (row > 0 && (animals[row - 1][col] == null || animals[row - 1][col].getColor() != turn)) {
validMove = true;
}
if (row < 3 && (animals[row + 1][col] == null || animals[row + 1][col].getColor() != turn)) {
validMove = true;
}
if (col > 0 && (animals[row][col - 1] == null || animals[row][col - 1].getColor() != turn)) {
validMove = true;
}
if (col < 3 && (animals[row][col + 1] == null || animals[row][col + 1].getColor() != turn)) {
validMove = true;
}
if (!validMove) {
return;
}
int choice = JOptionPane.showOptionDialog(null, "Select a target", "Move", JOptionPane.DEFAULT_OPTION, JOptionPane.PLAIN_MESSAGE, null, null, null);
if (choice == -1) {
return;
}
int targetRow = choice / 4, targetCol = choice % 4;
if (animals[targetRow][targetCol] != null && animals[targetRow][targetCol].getColor() == turn) {
return;
}
if (animals[row][col].compareRank(animals[targetRow][targetCol]) >= 0) {
if (animals[targetRow][targetCol] != null && animals[targetRow][targetCol].getType() == AnimalType.MOUSE) {
gameOver = true;
}
animals[targetRow][targetCol] = animals[row][col];
animals[row][col] = null;
turn = turn == AnimalColor.RED ? AnimalColor.BLUE : AnimalColor.RED;
updateButtons();
}
}
}
public void updateButtons() {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (animals[i][j] == null) {
buttons[i][j].setIcon(null);
} else {
ImageIcon icon = new ImageIcon(animals[i][j].getImageFile());
buttons[i][j].setIcon(icon);
}
}
}
}
public void resetGame() {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
animals[i][j] = null;
}
}
turn = AnimalColor.RED;
gameOver = false;
setupGame();
}
public static void shuffleArray(Object[] array) {
for (int i = 0; i < array.length; i++) {
int j = (int)(Math.random() * (array.length - i)) + i;
Object temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
public static void main(String[] args) {
AnimalGame game = new AnimalGame();
}
}
enum AnimalColor {
RED, BLUE
}
enum AnimalType {
ELEPHANT("elephant", 8),
LION("lion", 7),
TIGER("tiger", 6),
LEOPARD("leopard", 5),
WOLF("wolf", 4),
DOG("dog", 3),
CAT("cat", 2),
MOUSE("mouse", 1);
private String imageFile;
private int rank;
AnimalType(String imageFile, int rank) {
this.imageFile = imageFile;
this.rank = rank;
}
public String getImageFile() {
return imageFile;
}
public int getRank() {
return rank;
}
}
class Animal {
private AnimalType type;
private AnimalColor color;
public Animal(AnimalType type, AnimalColor color) {
this.type = type;
this.color = color;
}
public AnimalType getType() {
return type;
}
public AnimalColor getColor() {
return color;
}
public int compareRank(Animal other) {
return Integer.compare(this.type.getRank(), other.type.getRank());
}
public String getImageFile() {
return "images/" + color.toString().toLowerCase() + "_" + type.getImageFile() + ".png";
}
}
```
这个游戏使用了Java的GUI库Swing来创建界面。在游戏开始时,我们初始化一个4x4的网格,并在其中随机分配红色和蓝色的动物。每次玩家点击一个动物时,程序会弹出一个对话框,让玩家选择一个目标格子进行移动。如果目标格子中有动物,则会比较两个动物的大小,如果当前动物比目标动物大,则目标动物被吃掉。当任意一方的老鼠被吃掉时,游戏结束并提示玩家是否重新开始游戏。
阅读全文
相关推荐






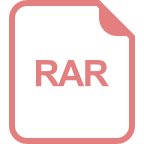

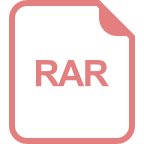
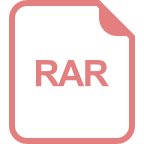

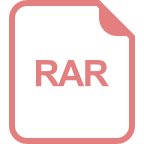
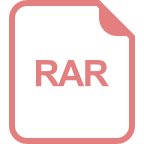
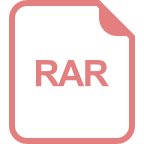




