如何使用Vue的ref属性隐藏特定的子组件?
时间: 2024-11-30 12:20:47 浏览: 22
在Vue中,你可以使用`v-if`指令结合`ref`属性来动态控制子组件的显示和隐藏。假设你有一个名为`myChildComponent`的子组件,首先确保在模板中给它分配了一个`ref`:
```html
<template>
<div>
<button @click="toggleVisibility">隐藏/显示子组件</button>
<my-child-component ref="childComp" v-if="showChild"></my-child-component>
</div>
</template>
```
然后,在你的Vue实例的`methods`对象中,编写一个`toggleVisibility`函数,通过改变`showChild`数据来切换子组件的可见性:
```javascript
export default {
data() {
return {
showChild: true,
};
},
methods: {
toggleVisibility() {
this.showChild = !this.showChild;
},
},
};
```
当`showChild`为`true`时,子组件会被显示;当`showChild`为`false`时,子组件会被隐藏。这样,每次点击按钮,都会更新`showChild`的状态,进而影响子组件的显示状态。
相关问题
vue2 用ref在子组件获取同级组件的的dom
在Vue 2中,`ref`是一个特殊的属性,用于创建一个响应式的引用到元素或者计算值。如果你想从子组件获取同级组件的DOM元素,你可以通过以下步骤:
1. 父组件里,首先需要声明一个`ref`,比如叫`myComponentRef`,并绑定给子组件:
```html
<template>
<div ref="myComponentRef">
<child-component :ref="childRef"></child-component>
</div>
</template>
<script>
export default {
components: { ChildComponent },
data() {
return {
childRef: null,
};
},
};
</script>
```
2. 在父组件的methods中,可以访问子组件的DOM:
```js
methods: {
getDOM() {
if (this.childRef) {
const childDOM = this.$refs.myComponentRef.$el.querySelector('[data-v-ref-child]');
// 这里假设子组件有一个带有"data-v-ref-child"的数据属性,如果不是,请替换为你实际的选择器
console.log(childDOM);
}
},
}
```
在这个例子中,`$el`属性返回的是当前元素的原始DOM节点,然后通过`querySelector`选择子组件的特定部分。
vue ref爷孙 组件通信
Vue中的组件通信有多种方式,其中一种常见的方式是使用`ref`属性进行爷孙组件之间的通信。
首先,在爷组件中,可以使用`ref`属性给子组件赋予一个特定的引用名。例如:
```vue
<template>
<div>
<child ref="childComponent"></child>
</div>
</template>
<script>
import Child from './Child.vue';
export default {
components: {
Child
},
mounted() {
console.log(this.$refs.childComponent); // 子组件的引用
}
}
</script>
```
然后,在孙组件中,可以通过`$parent`属性访问到爷组件的实例,并从中获取相应的数据或调用方法。例如:
```vue
<template>
<div>
<button @click="sendMessageToGrandpa">发送消息给爷爷</button>
</div>
</template>
<script>
export default {
methods: {
sendMessageToGrandpa() {
this.$parent.someMethodInGrandpa(); // 调用爷组件的方法
this.$parent.someDataInGrandpa = 'Hello Grandpa'; // 修改爷组件的数据
}
}
}
</script>
```
通过在爷组件中使用`ref`属性获取到子组件的引用,再通过`$parent`属性在孙组件中访问到爷组件,就可以实现爷孙组件之间的通信。请注意,这种方式只适用于爷组件直接包含孙组件的情况。如果组件层级很深,则会变得复杂,可以考虑使用其他更灵活的通信方式,如事件总线、Vuex 状态管理等。
阅读全文
相关推荐
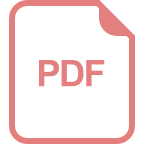
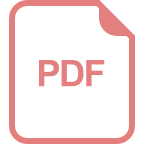
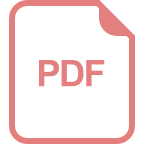
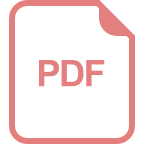












