通过kafka api消费指定时间范围内的kafka topic数据
时间: 2023-10-07 22:10:38 浏览: 60
您好!感谢您的提问。针对您的问题,我们可以通过使用 Kafka 的 Consumer API 来消费指定时间范围内的 Kafka Topic 数据。具体的实现方式包括以下几个步骤:
1. 创建 Kafka Consumer 对象
```
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("group.id", "test-group");
props.put("enable.auto.commit", "false");
props.put("auto.offset.reset", "earliest");
props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(props);
```
在这个示例代码中,我们创建了一个 Kafka Consumer 对象,并通过指定的参数来配置了该对象的一些属性,包括 Kafka 集群的连接地址、消费者组的 ID、是否自动提交消费位移、以及 Key 和 Value 的反序列化方法等。
2. 订阅 Kafka Topic
```
consumer.subscribe(Collections.singletonList("test-topic"), new ConsumerRebalanceListener() {
@Override
public void onPartitionsRevoked(Collection<TopicPartition> partitions) {
// 消费者失去了分区的所有权,需要处理分区的偏移量
}
@Override
public void onPartitionsAssigned(Collection<TopicPartition> partitions) {
// 消费者重新获得了分区的所有权,可以继续消费数据
}
});
```
在上述代码中,我们使用 Kafka Consumer 的 `subscribe()` 方法来订阅需要消费的 Topic,`ConsumerRebalanceListener` 则用于处理在分区发生变化时的情况,例如消费者失去了分区的所有权或重新获得了分区的所有权等。
3. 指定时间范围内的消费位移
```
Map<TopicPartition, Long> timestampToSearch = new HashMap<>();
for (TopicPartition partition : consumer.assignment()) {
timestampToSearch.put(partition, System.currentTimeMillis() - TimeUnit.MINUTES.toMillis(10));
}
Map<TopicPartition, OffsetAndTimestamp> offsetAndTimestamp = consumer.offsetsForTimes(timestampToSearch);
for (Map.Entry<TopicPartition, OffsetAndTimestamp> entry : offsetAndTimestamp.entrySet()) {
TopicPartition partition = entry.getKey();
OffsetAndTimestamp offsetTimestamp = entry.getValue();
if (offsetTimestamp != null) {
consumer.seek(partition, offsetTimestamp.offset());
}
}
```
在这个步骤中,我们创建了一个 `Map` 对象 `timestampToSearch`,并将消费者当前订阅的所有分区的消费位移时间戳都设置为 `System.currentTimeMillis() - TimeUnit.MINUTES.toMillis(10)` 表示过去 10 分钟以内的数据。然后,我们通过调用 Kafka Consumer 的 `offsetsForTimes()` 方法来获取指定时间戳之后的消费位移,最后再通过 `seek()` 方法将消费者定位到指定的消费位移处,即可开始消费指定时间范围内的 Kafka Topic 数据。
希望上述实现方式能够解决您的问题。如果您有其他的问题或疑问,请随时向我提问。
相关推荐
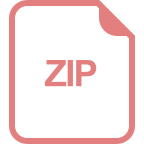
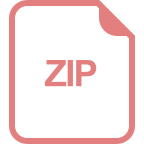














