修改代码,如何压缩保存图片?:screenshot = pyautogui.screenshot(screenshot_path)
时间: 2023-09-27 12:08:37 浏览: 305
你可以使用Pillow库来实现压缩并保存图片。需要先安装Pillow库,可以使用以下命令:
```
pip install Pillow
```
然后修改代码如下:
```
import pyautogui
from PIL import Image
# 设置截图保存路径
screenshot_path = 'screenshot.png'
# 执行截图操作
screenshot = pyautogui.screenshot()
# 压缩并保存截图
screenshot.save(screenshot_path, optimize=True, quality=75)
```
在保存时,我们可以设置 `optimize` 参数为 `True` 来启用压缩优化,同时可以设置 `quality` 参数来控制压缩质量。压缩后的图片文件大小会相应变小,但会有一定的损失。可以根据实际需求调整压缩质量。
相关问题
import cv2 import numpy as np import pyautogui import tkinter as tk from PIL import ImageTk, Image class WindowDetector: def __init__(self, template_path): self.template = cv2.imread(template_path, 0) self.w, self.h = self.template.shape[::-1] def detect(self): screenshot = pyautogui.screenshot() screenshot = np.array(screenshot) screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY) res = cv2.matchTemplate(screenshot, self.template, cv2.TM_CCOEFF_NORMED) threshold = 0.8 loc = np.where(res >= threshold) for pt in zip(*loc[::-1]): cv2.rectangle(screenshot, pt, (pt[0] + self.w, pt[1] + self.h), (0, 0, 255), 2) return screenshot class App: def __init__(self, template_path): self.window_detector = WindowDetector(template_path) self.root = tk.Tk() self.root.title("Window Detector") self.root.geometry("800x600") self.canvas = tk.Canvas(self.root, width=800, height=600) self.canvas.pack() self.template = ImageTk.PhotoImage(Image.open(template_path)) tk.Button(self.root, text="Detect", command=self.detect_window).pack() tk.Label(self.root, image=self.template).pack() self.root.mainloop() def detect_window(self): screenshot = self.window_detector.detect() img = ImageTk.PhotoImage(Image.fromarray(screenshot)) self.canvas.create_image(0, 0, anchor=tk.NW, image=img) self.root.update_idletasks() if __name__ == "__main__": app = App("template.png")
这段代码是用来检测屏幕上是否存在一个特定的窗口,它首先读取一个模板图像,然后使用pyautogui库截取屏幕图像。接着,它使用OpenCV中的模板匹配算法来在屏幕截图中寻找模板图像的匹配位置。如果匹配程度超过了设定的阈值,就会在屏幕截图中标出匹配的位置。最后,它使用tkinter库创建了一个GUI窗口,其中包含一个按钮和一个标签,用户可以点击按钮来检测屏幕上是否存在指定的窗口,并在标签中显示检测结果。
帮我优化我下面发送的代码,使图片识别过程中在屏幕上绘制出图片位置import tkinter as tk from tkinter import filedialog import pyautogui class Application(tk.Frame): def __init__(self, master=None): super().__init__(master) self.master = master self.pack() self.create_widgets() def create_widgets(self): self.select_button = tk.Button(self) self.select_button["text"] = "选择图片" self.select_button["command"] = self.select_image self.select_button.pack(side="top") self.result_label = tk.Label(self) self.result_label.pack(side="bottom") def select_image(self): # 弹出文件选择对话框选择要查找的图片 file_path = filedialog.askopenfilename(title="选择图片文件", filetypes=[("Image files", "*.png;*.jpg;*.jpeg")]) # 如果选择了图片则查找图片位置 if file_path: location = find_image_location(file_path) if location is not None: self.result_label["text"] = f"图片 {file_path} 的位置坐标为:{location}" else: self.result_label["text"] = f"图片 {file_path} 不存在于桌面" def find_image_location(image_path): # 加载图片并获取图片位置坐标 location = pyautogui.locateOnScreen(image_path,confidence=0.9) if location is not None: # 获取图片中心点的坐标 x, y = pyautogui.center(location) return (x, y) else: return None root = tk.Tk() app = Application(master=root) app.mainloop()
好的,你可以使用 pyautogui 库的 `screenshot()` 和 `drawRect()` 函数来实现在屏幕上绘制图片位置。
具体实现过程如下:
1. 在 find_image_location 函数中,在使用 pyautogui 库的 locateOnScreen 函数查找图片位置之前,先使用 pyautogui 库的 screenshot 函数获取当前屏幕的截图,并将其保存到变量 im 中。
2. 在使用 pyautogui 库的 locateOnScreen 函数查找图片位置之后,如果找到了图片,则使用 pyautogui 库的 drawRect 函数在屏幕上绘制出图片的位置矩形框。
3. 在使用 pyautogui 库的 drawRect 函数绘制矩形框时,需要传入图片位置的左上角坐标和右下角坐标,可以使用 pyautogui 库的 left、top、width 和 height 属性计算得到。
修改后的代码如下:
```
import tkinter as tk
from tkinter import filedialog
import pyautogui
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
self.select_button = tk.Button(self)
self.select_button["text"] = "选择图片"
self.select_button["command"] = self.select_image
self.select_button.pack(side="top")
self.result_label = tk.Label(self)
self.result_label.pack(side="bottom")
def select_image(self):
# 弹出文件选择对话框选择要查找的图片
file_path = filedialog.askopenfilename(title="选择图片文件", filetypes=[("Image files", "*.png;*.jpg;*.jpeg")])
# 如果选择了图片则查找图片位置
if file_path:
location = find_image_location(file_path)
if location is not None:
self.result_label["text"] = f"图片 {file_path} 的位置坐标为:{location}"
else:
self.result_label["text"] = f"图片 {file_path} 不存在于桌面"
def find_image_location(image_path):
# 获取当前屏幕截图
im = pyautogui.screenshot()
# 加载图片并获取图片位置坐标
location = pyautogui.locateOnScreen(image_path, confidence=0.9)
if location is not None:
# 获取图片位置的左上角和右下角坐标
left, top, width, height = location
right = left + width
bottom = top + height
# 在屏幕上绘制图片位置矩形框
pyautogui.drawRect(left, top, width, height)
# 获取图片中心点的坐标
x, y = pyautogui.center(location)
return (x, y)
else:
return None
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
这样,当程序找到图片位置时,会在屏幕上绘制出图片位置的矩形框,方便用户直观地了解图片位置。
阅读全文
相关推荐
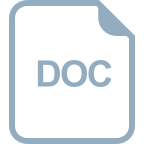
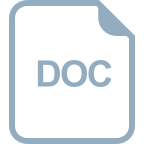
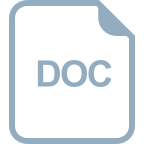













