python 贪吃蛇
时间: 2023-06-22 14:26:13 浏览: 108
好的,以下是一个简单的 Python 贪吃蛇游戏:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 定义窗口大小
window_width = 600
window_height = 400
# 创建游戏窗口
screen = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("Python 贪吃蛇游戏")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
# 定义贪吃蛇块的大小和初始位置
block_size = 10
snake_x = window_width / 2
snake_y = window_height / 2
# 定义贪吃蛇移动的初始方向
direction = "right"
# 定义食物的初始位置
food_x = round(random.randrange(0, window_width - block_size) / 10.0) * 10.0
food_y = round(random.randrange(0, window_height - block_size) / 10.0) * 10.0
# 定义贪吃蛇身体的初始长度和坐标列表
snake_length = 1
snake_list = []
# 定义游戏结束的变量
game_over = False
# 定义计分板
font_style = pygame.font.SysFont(None, 30)
def message(msg, color):
message = font_style.render(msg, True, color)
screen.blit(message, [window_width / 6, window_height / 3])
# 定义游戏循环
clock = pygame.time.Clock()
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
direction = "left"
elif event.key == pygame.K_RIGHT:
direction = "right"
elif event.key == pygame.K_UP:
direction = "up"
elif event.key == pygame.K_DOWN:
direction = "down"
# 移动贪吃蛇
if direction == "right":
snake_x += block_size
elif direction == "left":
snake_x -= block_size
elif direction == "up":
snake_y -= block_size
elif direction == "down":
snake_y += block_size
# 判断是否撞到边界
if snake_x >= window_width or snake_x < 0 or snake_y >= window_height or snake_y < 0:
game_over = True
# 绘制游戏窗口
screen.fill(white)
# 绘制食物
pygame.draw.rect(screen, green, [food_x, food_y, block_size, block_size])
# 绘制贪吃蛇身体
snake_head = []
snake_head.append(snake_x)
snake_head.append(snake_y)
snake_list.append(snake_head)
if len(snake_list) > snake_length:
del snake_list[0]
for x in snake_list[:-1]:
if x == snake_head:
game_over = True
for block in snake_list:
pygame.draw.rect(screen, black, [block[0], block[1], block_size, block_size])
# 判断是否吃到食物
if snake_x == food_x and snake_y == food_y:
food_x = round(random.randrange(0, window_width - block_size) / 10.0) * 10.0
food_y = round(random.randrange(0, window_height - block_size) / 10.0) * 10.0
snake_length += 1
# 绘制计分板
score = snake_length - 1
score_text = font_style.render("Score: " + str(score), True, black)
screen.blit(score_text, [0, 0])
# 刷新游戏窗口
pygame.display.update()
# 控制游戏速度
clock.tick(10)
# 关闭 Pygame
pygame.quit()
```
运行代码后,即可开始游戏。按方向键控制贪吃蛇的移动,吃到食物后贪吃蛇的长度会加一。如果贪吃蛇撞到边界或者碰到自己,则游戏结束。
阅读全文
相关推荐
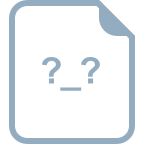


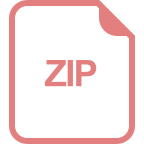


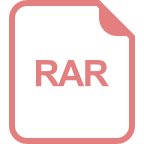
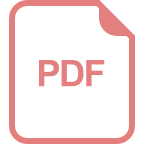
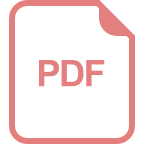
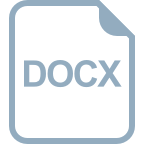
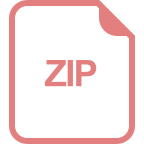