python 多线程,一个类中开启两个线程,使他们成为生产消费模式
时间: 2023-08-25 11:48:36 浏览: 81
可以使用Python的`threading`模块实现多线程生产消费模式。下面是一个示例代码,其中有一个类`ProducerConsumer`,它开启了两个线程,一个用于生产数据,另一个用于消费数据。
```python
import threading
import time
import random
from queue import Queue
class ProducerConsumer:
def __init__(self):
self.queue = Queue()
self.producer_thread = threading.Thread(target=self.produce)
self.consumer_thread = threading.Thread(target=self.consume)
def start(self):
self.producer_thread.start()
self.consumer_thread.start()
def produce(self):
while True:
# 模拟生产数据
data = random.randint(1, 100)
self.queue.put(data)
print(f"Produced: {data}")
time.sleep(random.random())
def consume(self):
while True:
# 模拟消费数据
data = self.queue.get()
print(f"Consumed: {data}")
self.queue.task_done()
time.sleep(random.random())
# 测试代码
pc = ProducerConsumer()
pc.start()
```
在上述代码中,`ProducerConsumer`类初始化时创建了一个`Queue`对象作为数据缓冲区。`produce`方法不断地生成随机数据并将其放入队列中,`consume`方法从队列中取出数据并进行消费。`start`方法启动了两个线程,分别执行`produce`和`consume`方法。
你可以根据实际需求修改`produce`和`consume`方法中的数据生成和消费逻辑。这个示例代码只是一个基本的多线程生产消费模式的实现,你可以根据自己的需求进行扩展和优化。
阅读全文
相关推荐












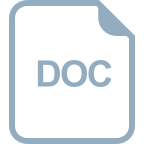





