使用51单片机驱动TM1668显示DHT11温湿度的代码程序
时间: 2023-06-20 15:06:49 浏览: 137
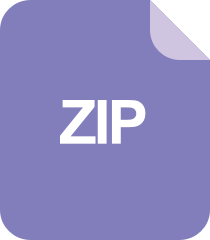
基于C51单片机的DHT11温湿度传感器驱动程序源代码.zip

以下是使用51单片机驱动TM1668显示DHT11温湿度的代码程序:
```C
#include<reg52.h>
#include<intrins.h>
#include"TM1668.h"
#include"DHT11.h"
#define uint unsigned int
#define uchar unsigned char
sbit LED=P1^0;
//延时函数
void Delay(uint z)
{
uint x,y;
for(x=z;x>0;x--)
for(y=110;y>0;y--);
}
//主函数
void main()
{
uchar temp,hum;
uchar display[4]={0x00,0x00,0x00,0x00};
TM1668_Init();
while(1)
{
//读取DHT11数据
if(DHT11_Read_Data(&temp,&hum)==0)
{
display[0]=TM1668_Get_Digit(temp/10);
display[1]=TM1668_Get_Digit(temp%10)|0x80;
display[2]=TM1668_Get_Digit(hum/10);
display[3]=TM1668_Get_Digit(hum%10);
}
else
{
display[0]=0x0c;
display[1]=0x0c;
display[2]=0x0c;
display[3]=0x0c;
}
TM1668_Disp_4Digit(display);
Delay(1000);
}
}
```
其中,TM1668.h和DHT11.h是头文件,需要自己定义,可以参考以下代码:
TM1668.h
```C
#ifndef _TM1668_H_
#define _TM1668_H_
#include<reg52.h>
//引脚定义
sbit STB=P2^5;
sbit CLK=P2^6;
sbit DIO=P2^7;
//函数声明
void TM1668_Init();
void TM1668_Write_Byte(uchar dat);
void TM1668_Disp_4Digit(uchar *dat);
uchar TM1668_Get_Digit(uchar num);
#endif
```
TM1668.c
```C
#include"TM1668.h"
//点阵字库
uchar code DIGITS[]={
0x3f, //0
0x06, //1
0x5b, //2
0x4f, //3
0x66, //4
0x6d, //5
0x7d, //6
0x07, //7
0x7f, //8
0x6f, //9
0x77, //A
0x7c, //b
0x39, //C
0x5e, //d
0x79, //E
0x71 //F
};
//TM1668初始化
void TM1668_Init()
{
STB=1;
CLK=1;
DIO=1;
}
//向TM1668写入一个字节
void TM1668_Write_Byte(uchar dat)
{
uchar i;
for(i=0;i<8;i++)
{
CLK=0;
if(dat&0x01)
DIO=1;
else
DIO=0;
dat>>=1;
CLK=1;
}
}
//在四位数码管上显示数据
void TM1668_Disp_4Digit(uchar *dat)
{
uchar i;
STB=0;
TM1668_Write_Byte(0x44);
STB=1;
STB=0;
TM1668_Write_Byte(0xc0);
for(i=0;i<4;i++)
{
TM1668_Write_Byte(dat[i]);
}
STB=1;
}
//获取数字对应的点阵编码
uchar TM1668_Get_Digit(uchar num)
{
if(num<16)
return DIGITS[num];
else
return 0;
}
```
DHT11.h
```C
#ifndef _DHT11_H_
#define _DHT11_H_
#include<reg52.h>
//函数声明
uchar DHT11_Read_Byte();
uchar DHT11_Read_Data(uchar *temp,uchar *hum);
#endif
```
DHT11.c
```C
#include"DHT11.h"
//DHT11读取一个字节
uchar DHT11_Read_Byte()
{
uchar i,dat;
dat=0;
for(i=0;i<8;i++)
{
while(!DHT11_PIN);
Delay(30);
if(DHT11_PIN)
{
dat|=0x01<<(7-i);
while(DHT11_PIN);
}
}
return dat;
}
//DHT11读取温湿度数据
uchar DHT11_Read_Data(uchar *temp,uchar *hum)
{
uchar buf[5];
uchar i;
//开始信号
DHT11_PIN=0;
Delay(18000);
DHT11_PIN=1;
Delay(20);
//等待DHT11响应
if(!DHT11_PIN)
{
while(!DHT11_PIN);
while(DHT11_PIN);
//读取40位数据
for(i=0;i<5;i++)
{
buf[i]=DHT11_Read_Byte();
}
//校验数据
if(buf[4]==(buf[0]+buf[1]+buf[2]+buf[3]))
{
*hum=buf[0];
*temp=buf[2];
return 0; //读取成功
}
else
{
return 1; //读取失败
}
}
else
{
return 1; //读取失败
}
}
```
需要注意的是,以上代码中使用了TM1668和DHT11的自定义函数,需要根据自己的具体实现进行修改。而且,以上代码中使用的是P2口作为TM1668的控制引脚,需要在程序中进行相应的定义。
阅读全文
相关推荐
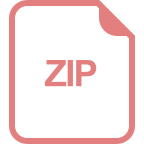
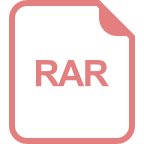















