讲解一下以下程序:#include<stdio.h> #include<stdlib.h> int shuzu()//数组方式 { int tao[10];//创立一个数组 tao[0]=1; //第10天剩1个桃子 int i; for(i=1;i<10;i++) tao[i]=(tao[i-1]+1)*2; printf("数组方法所求桃子总数:%d\n",tao[9]); return 0; } //递归方法 int f(int n) { if(n==10) { return 1;//结束循环 } else { return (f(n+1)+1)*2; } } //链数据结构 typedef struct tao { int count; //桃子个数 struct tao*next;// 建立结构体指针 }tao; int main() { tao*head=(tao*)malloc(sizeof(tao));//建立一个初始结构体 ,获得一个初始链式结构 head->count=1; //初始变量赋值 head->next=NULL;// int i=0; for(i=0;i<9;i++) { tao*newnode=(tao*)malloc(sizeof(tao));//建立一个结构体 ,获取结构空间 newnode->count=(head->count+1)*2; //当前桃子数量是前一天数量的一半加一 newnode->next=head;//指向头指针 head=newnode; } int sum=0; tao*current=head; sum=current->count; current=current->next; current=head; // 释放内存 while(current!=NULL) { tao*temp=current; current=current->next; free(temp); } shuzu(); printf("链表方法所求桃子总数:%d\n",sum); f(10); printf("递归方法所求桃子总数:%d\n",f(1)); return 0; }
时间: 2024-04-26 11:21:56 浏览: 7
这是一段 C 语言代码,主要实现了三种方法来计算猴子最初摘了多少个桃子,以及每天吃掉一半再多吃一个,最后剩下了一个桃子。程序分别采用了数组方式、递归方式和链表方式来实现。
在代码中,首先定义了一个数组 ta0,用来存储每天剩余的桃子数。然后通过循环计算每天的桃子数,最终输出第10天剩余的桃子数。
接着使用递归方式实现,函数 f(n) 表示第 n 天剩余的桃子数,通过递归调用 f(n+1) 来计算第 n 天的桃子数。当递归到第 10 天时,返回剩余的桃子数。最终输出第10天剩余的桃子数。
最后使用链表方式实现,定义了一个结构体 tao,其中 count 表示桃子的数量,next 表示下一个结构体的指针。通过循环创建一个包括 10 个结构体的链表,每个结构体的桃子数是前一天数量的一半加一。最终计算出第一天的桃子数,并输出第10天剩余的桃子数。
代码最后释放了链表结构体的内存空间,并调用了数组方式和递归方式的函数,分别输出第10天剩余的桃子数。
相关问题
include <stdio.h>#include <stdlib.h>
这是一个C语言的标准头文件的引用,其中<stdio.h>包含了C语言的输入输出函数,而<stdlib.h>包含了一些通用的工具函数和内存分配函数。这些头文件在C语言中非常常见,几乎每个C程序都会用到它们。下面是一个简单的例子,展示了如何使用这两个头文件。
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
char str[100];
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered %d.\n", num);
printf("Enter a string: ");
scanf("%s", str);
printf("You entered %s.\n", str);
return 0;
}
```
上面的程序使用了<stdio.h>中的printf和scanf函数,以及<stdlib.h>中的exit函数。输入一个整数和一个字符串,然后将它们输出到控制台上。
#include <stdio.h> #include <stdlib.h> typedef int ElemType; typedef struct LNode { ElemType da
`#include <stdio.h>`和`#include <stdlib.h>`是C语言中的两个头文件。`<stdio.h>`是标准输入输出头文件,提供了一些用于输入输出的函数和宏定义。`<stdlib.h>`是标准库头文件,提供了一些常用的函数和宏定义,如内存分配、随机数生成等。
在你的代码中,`typedef int ElemType;`定义了一个类型别名,将`int`类型命名为`ElemType`。
`typedef struct LNode`定义了一个结构体类型`LNode`,结构体是一种自定义的数据类型,可以包含多个不同类型的成员变量。在这里,`LNode`结构体中的成员变量还没有完整定义,因此代码截断了。
相关推荐
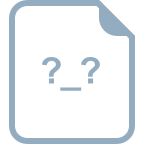
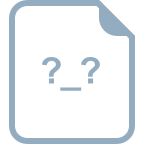













