用python统计字符个数包括英文数字空格和其他字符个数
时间: 2024-05-09 17:17:52 浏览: 74
可以使用Python内置的字符串函数来统计字符个数,具体代码如下:
```
string = input("请输入一个字符串:")
char_count = len(string) # 统计字符总数
letter_count = sum(1 for c in string if c.isalpha()) # 统计字母个数
digit_count = sum(1 for c in string if c.isdigit()) # 统计数字个数
space_count = sum(1 for c in string if c.isspace()) # 统计空格个数
other_count = char_count - letter_count - digit_count - space_count # 统计其他字符个数
print("总字符数:", char_count)
print("字母个数:", letter_count)
print("数字个数:", digit_count)
print("空格个数:", space_count)
print("其他字符个数:", other_count)
```
当然,如果您需要对英文字符进行大小写区分,可以使用`islower()`和`isupper()`函数来实现。
相关问题
用python统计其中英文字符、空格、数字和其他字符个数
### 回答1:
可以使用Python编写程序来统计一个字符串中英文字符、空格、数字和其他字符的个数。具体步骤如下:
1. 首先定义一个字符串,可以是一个变量或者直接在程序中写入字符串。
2. 然后使用Python内置函数isalpha()、isdigit()和isspace()来判断每个字符是英文字符、数字还是空格。
3. 使用for循环遍历字符串中的每个字符,将其分类并记录个数。
4. 最后输出每个分类的个数。
以下是一个示例程序:
```python
string = "Hello, World! 123"
count_en = 0
count_space = 0
count_digit = 0
count_other = 0
for char in string:
if char.isalpha():
count_en += 1
elif char.isspace():
count_space += 1
elif char.isdigit():
count_digit += 1
else:
count_other += 1
print("英文字符个数:", count_en)
print("空格个数:", count_space)
print("数字个数:", count_digit)
print("其他字符个数:", count_other)
```
输出结果为:
```
英文字符个数: 10
空格个数: 2
数字个数: 3
其他字符个数: 2
```
以上就是用Python统计字符串中英文字符、空格、数字和其他字符的方法。
### 回答2:
可以使用Python编写函数来统计给定字符串中英文字符、空格、数字和其他字符的个数。以下是一种可能的实现方法:
```python
def count_characters(string):
count_alpha = 0
count_digit = 0
count_space = 0
count_other = 0
for char in string:
if char.isalpha(): # 判断字符是否是英文字符
count_alpha += 1
elif char.isdigit(): # 判断字符是否是数字
count_digit += 1
elif char.isspace(): # 判断字符是否是空格
count_space += 1
else: # 其他字符
count_other += 1
return count_alpha, count_digit, count_space, count_other
# 测试函数
string = "Hello World! 123"
result = count_characters(string)
print("英文字符个数:", result[0])
print("数字个数:", result[1])
print("空格个数:", result[2])
print("其他字符个数:", result[3])
```
输出结果:
```
英文字符个数: 10
数字个数: 3
空格个数: 2
其他字符个数: 1
```
这段代码中定义了一个`count_characters`函数,接受一个字符串作为参数。然后,使用循环遍历字符串的每个字符,并使用`isalpha()`、`isdigit()`和`isspace()`等内置方法来判断字符的类型,从而进行统计。最后,返回各类型字符的个数,并进行打印输出。
### 回答3:
要实现这个功能,我们可以使用Python的字符串处理函数和一些控制流。
首先,我们需要记录英文字符数、空格数、数字数和其他字符数的计数器。
然后,通过遍历字符串中的每一个字符,通过判断字符的类型来将计数器相应地递增。
以下是一个简单的实现:
```python
def count_characters(string):
english_count = 0
space_count = 0
digit_count = 0
other_count = 0
for char in string:
if char.isalpha():
english_count += 1
elif char.isspace():
space_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
return english_count, space_count, digit_count, other_count
input_string = input("请输入一个字符串:")
english, space, digit, other = count_characters(input_string)
print("英文字母个数:", english)
print("空格个数:", space)
print("数字个数:", digit)
print("其他字符个数:", other)
```
这段代码首先定义了一个`count_characters`函数,它接受一个字符串作为输入。函数内部,我们使用四个计数器变量来保存各个类型字符的数量。然后,通过循环遍历字符串中的每一个字符,根据字符的类型将相应的计数器递增。
最后,我们在主程序中获取用户输入的字符串,并调用`count_characters`函数来获取各个类型字符的数量,然后打印出统计结果。
希望这能帮到你!
统计字符数字空格和其他字符个数
统计字符数字空格和其他字符个数通常是在文本处理或编程任务中常见的需求。这个过程可以分为几个步骤:
1. **遍历字符串**:首先,你需要获取输入字符串,并通过循环逐个检查每个字符。
2. **判断字符类别**:对于每一个字符,你可以检查它是否是数字、字母(包括中文字符)、空格,还是其他特殊字符。这通常通过ASCII码或者Unicode编码来识别。
- 数字:使用`isdigit()`函数(Python)或正则表达式`\d`(如JavaScript)检测。
- 空格:直接比较字符是否等于空格(如' ')。
- 其他字符:其余不是数字也不是空格的字符。
3. **计数**:对每种类型的字符创建一个计数器,每次遇到相应类型的字符就增加对应的计数。
4. **汇总结果**:最后,返回四种类型的计数值:数字字符数、空格数、非数字非空格字符数。
如果你需要具体的例子代码,我可以提供一个简单的示例:
```python
def count_chars(text):
num_count = sum(c.isdigit() for c in text)
space_count = text.count(' ')
other_count = len(text) - (num_count + space_count)
return num_count, space_count, other_count
text = "这是一个包含123空格和标点符号的字符串"
nums, spaces, others = count_chars(text)
print(f"数字:{nums}, 空格:{spaces}, 其他:{others}")
```
阅读全文
相关推荐
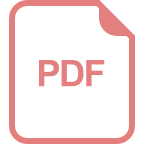
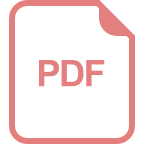
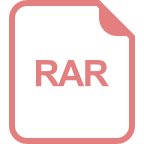












