用简单的python需要统计一个字符串字符个数英文数字空格和其他字符个数
时间: 2023-12-25 07:48:03 浏览: 48
可以使用Python中的isalpha()、isdigit()和isspace()等方法进行判断字符类型,然后统计各种类型的字符数量即可。以下是代码示例:
```
string = input("请输入一个字符串:")
letter_count = 0
digit_count = 0
space_count = 0
other_count = 0
for char in string:
if char.isalpha():
letter_count += 1
elif char.isdigit():
digit_count += 1
elif char.isspace():
space_count += 1
else:
other_count += 1
print("英文字母个数为:", letter_count)
print("数字个数为:", digit_count)
print("空格个数为:", space_count)
print("其他字符个数为:", other_count)
```
当然,这只是一种简单的方法,还有很多其他的实现方式。
相关问题
用Python定义一个函数输入一行字符串分别统计其中英文字母空格数字和其他字符个数英文字母空格数字和其他字符的个数
好的,我理解了你的要求。下面是用 Python 实现的函数,可以对输入的字符串进行统计:
```python
def count_chars(s):
# 初始化计数器
num_alpha = 0 # 英文字母个数
num_space = 0 # 空格个数
num_digit = 0 # 数字个数
num_other = 0 # 其他字符个数
# 遍历字符串中的每一个字符
for c in s:
if c.isalpha(): # 判断是否为英文字母
num_alpha += 1
elif c.isspace(): # 判断是否为空格
num_space += 1
elif c.isdigit(): # 判断是否为数字
num_digit += 1
else: # 其他字符
num_other += 1
# 输出统计结果
print("英文字母个数:", num_alpha)
print("空格个数:", num_space)
print("数字个数:", num_digit)
print("其他字符个数:", num_other)
```
你可以调用这个函数,把你想要统计的字符串作为参数传入即可,比如:
```python
count_chars("Hello 123 world!")
```
这样就能输出统计结果了。
python编程:对一行字符串统计不同字符个数,分别统计并输出中英文字符、空格、数字和其他字符个数。 输入格式: 一行字符串 输出格式: 依次输出中英文字符、空格、数字、和其他字符个数。
好的,这个问题我可以回答。可以使用Python的内置函数isalpha()、isdigit()和isspace()来判断字符的类型,然后进行统计。代码如下:
```
s = input()
zh_count = en_count = space_count = digit_count = other_count = 0
for c in s:
if c.isalpha():
if c.encode('utf-8').isalpha():
en_count += 1
else:
zh_count += 1
elif c.isspace():
space_count += 1
elif c.isdigit():
digit_count += 1
else:
other_count += 1
print(zh_count, en_count, space_count, digit_count, other_count)
```
其中,encode('utf-8').isalpha()用来判断是否为中文字符。
阅读全文
相关推荐
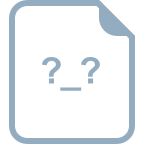
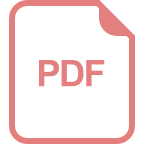













