python将图片保存到指定路径,并且格式为.dat(.bin)
时间: 2024-05-13 15:14:42 浏览: 6
你可以使用Pillow库来读取和保存图片,然后使用numpy库将图像转换为二进制数据并将其保存为.dat(.bin)文件。下面是Python代码示例:
```python
from PIL import Image
import numpy as np
# 读取图片
img = Image.open('example.jpg')
# 转换为灰度图像
img = img.convert('L')
# 将图像转换为numpy数组
img_arr = np.array(img)
# 将numpy数组转换为二进制数据
binary_data = img_arr.tobytes()
# 将二进制数据保存到.dat文件中
with open('example.dat', 'wb') as f:
f.write(binary_data)
```
这个例子中,我们首先使用Pillow库读取了一张图像,并将其转换为灰度图像。然后,我们将图像转换为numpy数组,并使用numpy的tobytes方法将其转换为二进制数据。最后,我们将二进制数据写入到.dat文件中。
相关问题
python opencv以.dat(.bin)格式输出图片
首先,可以使用OpenCV库中的imread函数读取图像文件,并将其存储为numpy数组:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
```
然后,可以使用numpy中的tofile函数将numpy数组保存为二进制文件:
```python
img.tofile('image.dat')
```
如果想要将图像保存为.bin文件,可以使用以下代码:
```python
with open('image.bin', 'wb') as f:
f.write(img.tobytes())
```
注意,在读取二进制文件时,需要指定numpy数组的dtype和shape信息:
```python
with open('image.bin', 'rb') as f:
img_data = np.frombuffer(f.read(), dtype=np.uint8)
img = img_data.reshape((height, width, channels))
```
用image.save将图片保存到指定路径
可以使用PIL库中的Image模块来实现将图片保存到指定路径的操作,具体步骤如下:
1.导入PIL库中的Image模块
```python
from PIL import Image
```
2.打开需要保存的图片
```python
img = Image.open('image.jpg')
```
3.将图片保存到指定路径
```python
img.save('path/to/save/image.jpg')
```
完整代码示例如下:
```python
from PIL import Image
img = Image.open('image.jpg')
img.save('path/to/save/image.jpg')
```
其中,'image.jpg'是需要保存的图片路径,'path/to/save/image.jpg'是保存后的路径,可以根据需要进行修改。
相关推荐
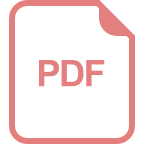












