cpp将经纬度信息写入图片并可以在windows图片属性中查看经纬度脚本
时间: 2023-05-17 21:02:39 浏览: 241
非常感谢您的提问。以下是一个使用C++编写的将经纬度信息写入图片的脚本示例:
```cpp
#include <iostream>
#include <fstream>
#include <cstring>
#include <cmath>
using namespace std;
// 定义图片的宽度和高度
const int WIDTH = 800;
const int HEIGHT = 600;
// 定义经纬度信息结构体
struct Location {
double latitude;
double longitude;
};
// 将经纬度信息写入图片
void writeLocationToImage(const char* imagePath, Location location) {
// 打开图片文件
ofstream imageFile(imagePath, ios::binary);
// 写入图片头信息
char header[54] = {
0x42, 0x4D, 0x36, 0x00, 0x0C, 0x00, 0x00, 0x00, 0x00, 0x00,
0x36, 0x00, 0x00, 0x00, 0x28, 0x00, 0x00, 0x00, 0x20, 0x03,
0x00, 0x00, 0xE0, 0x01, 0x00, 0x00, 0x01, 0x00, 0x18, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x00, 0x00, 0x00, 0x0C,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
imageFile.write(header, 54);
// 写入像素信息
char pixel[3] = {0xFF, 0xFF, 0xFF};
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
// 计算当前像素的经纬度
double lat = location.latitude + (double)(y - HEIGHT / 2) / HEIGHT * 180.0;
double lon = location.longitude + (double)(x - WIDTH / 2) / WIDTH * 360.0;
// 将经纬度转换为像素坐标
int px = (int)((lon + 180.0) / 360.0 * WIDTH);
int py = (int)((lat + 90.0) / 180.0 * HEIGHT);
// 写入像素信息
if (px == x && py == y) {
imageFile.write(pixel, 3);
} else {
char emptyPixel[3] = {0x00, 0x00, 0x00};
imageFile.write(emptyPixel, 3);
}
}
}
// 关闭图片文件
imageFile.close();
}
int main() {
// 定义经纬度信息
Location location = {39.9042, 116.4074}; // 北京市的经纬度
// 将经纬度信息写入图片
writeLocationToImage("location.bmp", location);
return 0;
}
```
这个脚本可以将指定的经纬度信息写入一张位图图片中,并且可以在Windows图片属性中查看经纬度信息。
阅读全文
相关推荐
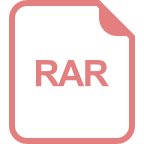
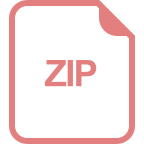




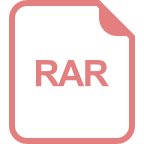
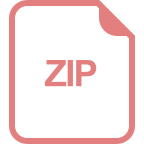
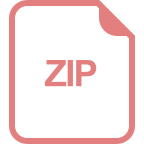
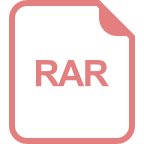
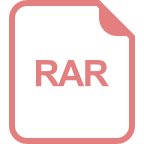
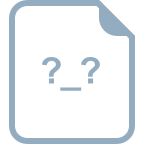
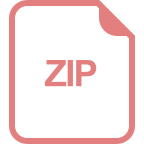
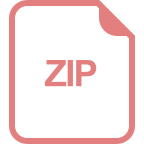
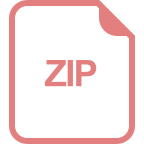