messagebox弹出框 输入框为密码框时 加一个眼睛
时间: 2024-03-09 15:45:04 浏览: 16
你可以在 `messageBox` 的 `inputType` 属性中设置为 `'password'`,这样会显示密码框。然后,你可以在弹框中添加一个按钮,通过点击按钮来切换密码框的显示方式。下面是一个示例代码:
```javascript
<template>
<div>
<el-button type="primary" @click="openMsgbox">打开弹框</el-button>
</div>
</template>
<script>
export default {
data() {
return {
isShowPassword: false, // 是否显示密码
password: '' // 输入的密码
}
},
methods: {
openMsgbox() {
this.$msgbox({
title: '请输入密码',
inputType: 'password',
inputPattern: /^[^\s\u4e00-\u9fa5]{6,20}(?=.*[A-Za-z])(?=.*\d)(?=.*[~!@#$%^&*()_+{}:"<>?\`\-=[\]\\;',./])/,
inputErrorMessage: '密码格式不正确',
showCancelButton: true,
confirmButtonText: '确定',
cancelButtonText: '取消',
customClass: 'message-box-password'
}).then(val => {
this.password = val;
}).catch(() => {
console.log('取消输入');
});
},
toggleShowPassword() {
this.isShowPassword = !this.isShowPassword;
}
}
}
</script>
<style>
.message-box-password .el-input__suffix-inner {
cursor: pointer;
}
</style>
```
在这段代码中,我们在弹框的 `customClass` 属性中指定了一个样式类名,这个样式类名用于控制密码框的样式。同时,我们在弹框中添加了一个按钮,按钮的样式可以通过样式类名来控制。
下面是这个样式类名的样式代码:
```css
.message-box-password .el-input__suffix-inner {
cursor: pointer;
}
```
这段代码的含义是:当弹框的样式类名为 `message-box-password` 时,将密码框后面的图标的鼠标指针设置为手形,表示可以点击。
然后,我们需要在弹框中添加一个按钮,用于切换密码框的显示方式。在弹框的 `beforeClose` 属性中添加一个函数,用于在弹框关闭之前执行一些操作。下面是修改后的代码:
```javascript
openMsgbox() {
this.$msgbox({
title: '请输入密码',
inputType: 'password',
inputPattern: /^[^\s\u4e00-\u9fa5]{6,20}(?=.*[A-Za-z])(?=.*\d)(?=.*[~!@#$%^&*()_+{}:"<>?\`\-=[\]\\;',./])/,
inputErrorMessage: '密码格式不正确',
showCancelButton: true,
confirmButtonText: '确定',
cancelButtonText: '取消',
customClass: 'message-box-password',
beforeClose: (action, instance, done) => {
if (action === 'confirm') {
this.password = instance.inputValue;
}
done();
}
});
},
```
在 `beforeClose` 函数中,我们首先判断用户点击的是确定按钮还是取消按钮。如果点击的是确定按钮,就将密码赋值给组件的 `password` 属性。然后,调用 `done()` 函数来关闭弹框。
接下来,我们需要在弹框中添加一个按钮,用于切换密码框的显示方式。在弹框的 `inputIcon` 属性中添加一个函数,用于设置密码框后面的图标。下面是修改后的代码:
```javascript
openMsgbox() {
this.$msgbox({
title: '请输入密码',
inputType: 'password',
inputPattern: /^[^\s\u4e00-\u9fa5]{6,20}(?=.*[A-Za-z])(?=.*\d)(?=.*[~!@#$%^&*()_+{}:"<>?\`\-=[\]\\;',./])/,
inputErrorMessage: '密码格式不正确',
showCancelButton: true,
confirmButtonText: '确定',
cancelButtonText: '取消',
customClass: 'message-box-password',
beforeClose: (action, instance, done) => {
if (action === 'confirm') {
this.password = instance.inputValue;
}
done();
},
inputIcon: (h, { isShowPassword }) => {
return h('i', {
class: ['el-input__icon', 'el-icon-view', isShowPassword ? 'active' : ''],
on: {
click: () => {
this.toggleShowPassword();
}
}
});
}
});
},
```
在 `inputIcon` 函数中,我们首先判断当前密码框的显示方式是否为明文。如果是明文,就给图标添加一个 `active` 样式类名,表示当前密码框的显示方式为明文。然后,给图标添加一个 `click` 事件,用于切换密码框的显示方式。在事件处理函数中,调用 `toggleShowPassword` 函数来切换密码框的显示方式。
最后,我们需要在组件中添加 `toggleShowPassword` 函数,用于切换密码框的显示方式。下面是完整的代码:
```javascript
<template>
<div>
<el-button type="primary" @click="openMsgbox">打开弹框</el-button>
</div>
</template>
<script>
export default {
data() {
return {
isShowPassword: false, // 是否显示密码
password: '' // 输入的密码
}
},
methods: {
openMsgbox() {
this.$msgbox({
title: '请输入密码',
inputType: 'password',
inputPattern: /^[^\s\u4e00-\u9fa5]{6,20}(?=.*[A-Za-z])(?=.*\d)(?=.*[~!@#$%^&*()_+{}:"<>?\`\-=[\]\\;',./])/,
inputErrorMessage: '密码格式不正确',
showCancelButton: true,
confirmButtonText: '确定',
cancelButtonText: '取消',
customClass: 'message-box-password',
beforeClose: (action, instance, done) => {
if (action === 'confirm') {
this.password = instance.inputValue;
}
done();
},
inputIcon: (h, { isShowPassword }) => {
return h('i', {
class: ['el-input__icon', 'el-icon-view', isShowPassword ? 'active' : ''],
on: {
click: () => {
this.toggleShowPassword();
}
}
});
}
});
},
toggleShowPassword() {
this.isShowPassword = !this.isShowPassword;
}
}
}
</script>
<style>
.message-box-password .el-input__suffix-inner {
cursor: pointer;
}
.message-box-password .el-input__icon.el-icon-view.active {
color: #409EFF;
}
</style>
```
在这段代码中,我们通过添加一个按钮来切换密码框的显示方式。这个按钮的样式可以通过样式类名来控制。同时,我们在弹框中添加了一个 `beforeClose` 函数,用于在弹框关闭之前执行一些操作。在 `beforeClose` 函数中,我们判断用户点击的是确定按钮还是取消按钮,并将密码赋值给组件的 `password` 属性。最后,我们在弹框中添加了一个 `inputIcon` 函数,用于设置密码框后面的图标。在这个函数中,我们判断当前密码框的显示方式,并添加一个 `click` 事件,用于切换密码框的显示方式。
相关推荐
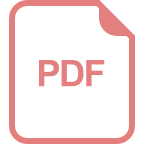
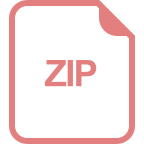














