用jwt写一个非对称加密生成token的代码
时间: 2023-11-30 19:04:03 浏览: 35
非对称加密是一种加密方式,它使用公钥和私钥来加密和解密数据。JWT(JSON Web Token)是一种用于认证和授权的标准,它通常使用对称加密算法来生成token。但是,我们也可以使用非对称加密算法来生成JWT token。下面是一个使用RSA非对称加密算法生成JWT token的代码示例:
```python
import jwt
import datetime
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import serialization, hashes
# 生成RSA公钥和私钥
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
# 把公钥和私钥保存到文件中
with open('private.pem', 'wb') as f:
f.write(private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.PKCS8,
encryption_algorithm=serialization.NoEncryption()
))
with open('public.pem', 'wb') as f:
f.write(public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
))
# 生成JWT token
payload = {
'user_id': 123,
'exp': datetime.datetime.utcnow() + datetime.timedelta(days=1)
}
# 把payload加密成JWT token
token = jwt.encode(
payload,
private_key,
algorithm='RS256'
)
# 验证JWT token
decoded_payload = jwt.decode(
token,
public_key,
algorithms=['RS256'],
options={'verify_exp': True}
)
print(decoded_payload)
```
这段代码使用了Python的cryptography库来生成RSA公钥和私钥,并使用jwt库来生成和验证JWT token。在生成JWT token时,我们使用了private_key参数来指定私钥,algorithm参数来指定使用的加密算法为RS256。在验证JWT token时,我们使用了public_key参数来指定公钥,algorithms参数来指定使用的加密算法为RS256,options参数中的verify_exp参数来指定是否验证token是否过期。
相关推荐
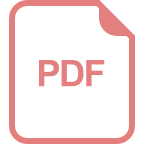
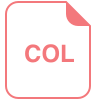
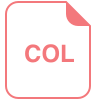
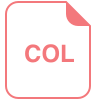
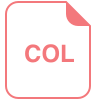
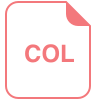









