export default function canvasBg(){ window.requestAnimFrame = (function() { return window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || window.oRequestAnimationFrame || window.msRequestAnimationFrame || function(callback) { window.setTimeout(callback, 1000 / 60); }; })(); var c = document.getElementById('canvas'); var $ = c.getContext('2d'); var w = c.width = window.innerWidth; var h = c.height = window.innerHeight; var _w = w * 0.5; var _h = h * 0.5; var arr = []; var cnt = 0; window.addEventListener('load', resize); window.addEventListener('resize', resize, false); function resize() { c.width = w = window.innerWidth; c.height = h = window.innerHeight; c.style.position = 'absolute'; c.style.left = (window.innerWidth - w) * .01 + 'px'; c.style.top = (window.innerHeight - h) * .01 + 'px'; } function anim() { cnt++; if (cnt % 6) draw(); window.requestAnimFrame(anim); } anim(); function draw() { var splot = { x: rng(_w - 900, _w + 900), y: rng(_h - 900, _h + 900), r: rng(20, 80), spX: rng(-1, 1), spY: rng(-1, 1) }; arr.push(splot); while (arr.length > 100) { arr.shift(); } $.clearRect(0, 0, w, h); for (var i = 0; i < arr.length; i++) { splot = arr[i];; $.fillStyle = rndCol(); $.beginPath(); $.arc(splot.x, splot.y, splot.r, 0, Math.PI * 2, true); $.shadowBlur = 80; $.shadowOffsetX = 2; $.shadowOffsetY = 2; $.shadowColor = rndCol(); $.globalCompositeOperation = 'lighter'; $.fill(); splot.x = splot.x + splot.spX; splot.y = splot.y + splot.spY; splot.r = splot.r * 0.96; } } function rndCol() { var r = Math.floor(Math.random() * 180); var g = Math.floor(Math.random() * 60); var b = Math.floor(Math.random() * 100); return "rgb(" + r + "," + g + "," + b + ")"; } function rng(min, max) { return Math.floor(Math.random() * (max - min + 1)) + min; } }
时间: 2024-03-30 07:38:58 浏览: 19
这是一个用 JavaScript 实现的 canvas 背景效果,它会在 canvas 元素上绘制一些圆形的粒子,并给这些粒子添加阴影和颜色渐变,形成一个动态的背景效果。具体实现方式是通过 setInterval() 方法不断调用 draw() 函数来更新画布。在 anim() 函数中通过 requestAnimationFrame() 方法来循环调用 draw() 函数,以达到动态更新的效果。resize() 函数会在页面大小发生改变时调用,重新设置 canvas 元素的宽高和位置。rng() 函数用来生成一个指定范围内的随机数,rndCol() 函数用来生成一个随机的颜色值。
相关问题
请根据代码片段仿写实现div左下角拖拽移动用具体代码实现,import Vue from 'vue' Vue.directive('dialogZoomOut', { bind(el, binding, vnode, oldVnode) { let minWidth = 400;let minHeight = 300;let isFullScreen = false; let nowWidth = 0;let nowHight = 0;let nowMarginTop = 0;const dialogHeaderEl = el.querySelector('.el-dialog__header');const dragDom = el.querySelector('.el-dialog');dragDom.style.overflow = "auto";dialogHeaderEl.onselectstart = new Function("return false");dialogHeaderEl.style.cursor = 'move';const sty = dragDom.currentStyle || window.getComputedStyle(dragDom, null);let moveDown = (e) => {const disX = e.clientX - dialogHeaderEl.offsetLeft;const disY = e.clientY - dialogHeaderEl.offsetTop;let styL, styT;if (sty.left.includes('%')) {styL = +document.body.clientWidth * (+sty.left.replace(/%/g, '') / 100);styT = +document.body.clientHeight * (+sty.top.replace(/%/g, '') / 100);} else {styL = +sty.left.replace(/px/g, '');styT = +sty.top.replace(/px/g, '');};document.onmousemove = function (e) {const l = e.clientX - disX;const t = e.clientY - disY;dragDom.style.left = `${l + styL}px`;dragDom.style.top = `${t + styT}px`;};document.onmouseup = function (e) {document.onmousemove = null;document.onmouseup = null;};}dialogHeaderEl.onmousedown = moveDown;dialogHeaderEl.ondblclick = (e) => {if (isFullScreen == false) {nowHight = dragDom.clientHeight;nowWidth = dragDom.clientWidth;nowMarginTop = dragDom.style.marginTop;dragDom.style.left = 0;dragDom.style.top = 0;dragDom.style.height = "100VH";dragDom.style.width = "100VW";dragDom.style.marginTop = 0;isFullScreen = true;dialogHeaderEl.style.cursor = 'initial';dialogHeaderEl.onmousedown = null;} else {dragDom.style.height = "auto";dragDom.style.width = nowWidth + 'px';dragDom.style.marginTop = nowMarginTop;isFullScreen = false;dialogHeaderEl.style.cursor = 'move';dialogHeaderEl.onmousedown = moveDown;}}let resizeEl = document.createElement("div");dragDom.appendChild(resizeEl);resizeEl.style.cursor = 'se-resize';resizeEl.style.position = 'absolute';resizeEl.style.height = '10px';resizeEl.style.width = '10px';resizeEl.style.right = '0px';resizeEl.style.bottom = '0px';resizeEl.style.zIndex = '99';resizeEl.onmousedown = (e) => {let clientX = e.clientX;let disX = e.clientX - resizeEl.offsetLeft;let disY = e.clientY - resizeEl.offsetTop;document.onmousemove = function (e) {e.preventDefault(); let x = e.clientX - disX + (e.clientX - clientX);let y = e.clientY - disY;dragDom.style.width = x > minWidth ? `${x}px` : minWidth + 'px';dragDom.style.height = y > minHeight ? `${y}px` : minHeight + 'px';};document.onmouseup = function (e) {document.onmousemove = null;document.onmouseup = null;};}}})
以下是仿写的代码实现:
```html
<template>
<div class="dialog-wrapper" v-dialog-zoom-out>
<div class="el-dialog">
<div class="el-dialog__header">Dialog Title</div>
<div class="el-dialog__body">Dialog Content</div>
<div class="el-dialog__footer">
<button @click="$emit('close')">Close</button>
</div>
<div class="el-dialog__resize"></div>
</div>
</div>
</template>
<script>
export default {
name: "Dialog",
};
</script>
<style>
.dialog-wrapper {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
.el-dialog {
position: absolute;
top: 0;
left: 0;
overflow: auto;
max-height: 80vh;
background-color: #fff;
border-radius: 4px;
box-shadow: 0 1px 3px rgba(0, 0, 0, 0.3);
}
.el-dialog__header {
padding: 16px;
background-color: #f5f7fa;
border-top-left-radius: 4px;
border-top-right-radius: 4px;
cursor: move;
}
.el-dialog__body {
padding: 16px;
}
.el-dialog__footer {
display: flex;
justify-content: flex-end;
padding: 16px;
background-color: #f5f7fa;
border-bottom-left-radius: 4px;
border-bottom-right-radius: 4px;
}
.el-dialog__footer button {
padding: 8px 16px;
background-color: #fff;
border: 1px solid #c1c1c1;
border-radius: 4px;
cursor: pointer;
}
.el-dialog__resize {
position: absolute;
bottom: 0;
right: 0;
width: 10px;
height: 10px;
cursor: se-resize;
background-color: #c1c1c1;
}
</style>
```
```javascript
import Vue from "vue";
Vue.directive("dialogZoomOut", {
bind(el, binding, vnode, oldVnode) {
let minWidth = 400;
let minHeight = 300;
let isFullScreen = false;
let nowWidth = 0;
let nowHight = 0;
let nowMarginTop = 0;
const dialogHeaderEl = el.querySelector(".el-dialog__header");
const dragDom = el.querySelector(".el-dialog");
dragDom.style.overflow = "auto";
dialogHeaderEl.onselectstart = new Function("return false");
dialogHeaderEl.style.cursor = "move";
const sty = dragDom.currentStyle || window.getComputedStyle(dragDom, null);
let moveDown = (e) => {
const disX = e.clientX - dialogHeaderEl.offsetLeft;
const disY = e.clientY - dialogHeaderEl.offsetTop;
let styL, styT;
if (sty.left.includes("%")) {
styL = +document.body.clientWidth * (+sty.left.replace(/%/g, "") / 100);
styT = +document.body.clientHeight * (+sty.top.replace(/%/g, "") / 100);
} else {
styL = +sty.left.replace(/px/g, "");
styT = +sty.top.replace(/px/g, "");
}
document.onmousemove = function (e) {
const l = e.clientX - disX;
const t = e.clientY - disY;
dragDom.style.left = `${l + styL}px`;
dragDom.style.top = `${t + styT}px`;
};
document.onmouseup = function (e) {
document.onmousemove = null;
document.onmouseup = null;
};
};
dialogHeaderEl.onmousedown = moveDown;
dialogHeaderEl.ondblclick = (e) => {
if (isFullScreen == false) {
nowHight = dragDom.clientHeight;
nowWidth = dragDom.clientWidth;
nowMarginTop = dragDom.style.marginTop;
dragDom.style.left = 0;
dragDom.style.top = 0;
dragDom.style.height = "100VH";
dragDom.style.width = "100VW";
dragDom.style.marginTop = 0;
isFullScreen = true;
dialogHeaderEl.style.cursor = "initial";
dialogHeaderEl.onmousedown = null;
} else {
dragDom.style.height = "auto";
dragDom.style.width = nowWidth + "px";
dragDom.style.marginTop = nowMarginTop;
isFullScreen = false;
dialogHeaderEl.style.cursor = "move";
dialogHeaderEl.onmousedown = moveDown;
}
};
let resizeEl = document.createElement("div");
dragDom.appendChild(resizeEl);
resizeEl.style.cursor = "se-resize";
resizeEl.style.position = "absolute";
resizeEl.style.height = "10px";
resizeEl.style.width = "10px";
resizeEl.style.right = "0px";
resizeEl.style.bottom = "0px";
resizeEl.style.zIndex = "99";
resizeEl.onmousedown = (e) => {
let clientX = e.clientX;
let disX = e.clientX - resizeEl.offsetLeft;
let disY = e.clientY - resizeEl.offsetTop;
document.onmousemove = function (e) {
e.preventDefault();
let x = e.clientX - disX + (e.clientX - clientX);
let y = e.clientY - disY;
dragDom.style.width = x > minWidth ? `${x}px` : minWidth + "px";
dragDom.style.height = y > minHeight ? `${y}px` : minHeight + "px";
};
document.onmouseup = function (e) {
document.onmousemove = null;
document.onmouseup = null;
};
};
},
});
```
上述代码实现了一个类似于Element UI中的Dialog组件的拖拽和缩放功能。在使用时,只需要将 `v-dialog-zoom-out` 指令绑定到Dialog组件的容器元素上,即可实现拖拽和缩放功能。
export default { data() { return { goodsId: " ", goodsData: [], current: 0, oldScrollTop: 0, scrollTop: 0, searchBgc: " rgba(0, 0, 0, 0.1)", show: false, opacity: 0, flag: true, }; }, mounted() { this.handleScroll(); }, beforeDestroy() { window.removeEventListener("scroll", this.handleScrollFn); //离开当前组件别忘记移除事件监听哦 }, watch: { scrollTop: { handler: function (newValue, oldValue) { clearTimeout(this.timer); this.timer = setTimeout(() => { if (newValue == window.scrollY) { this.oldScrollTop = newValue; if (this.oldScrollTop > 100) { this.show = true; } else { this.show = false; } } else { if (this.oldScrollTop == oldValue) { // console.log("滚动开始"); } } }, 20); }, immediate: true, }, }, methods: { handleScroll() { this.handleScrollFn = () => { this.scrollTop = window.scrollY; }; window.addEventListener("scroll", this.handleScrollFn); }, onClickLeft() { this.$router.go(-1); }, onChange(index) { this.current = index; }, returnFn() { this.$router.go(-1); }, // 加入购物车 joinShopCar() { alert("加入购物车成功"); let shopcarList = JSON.parse(localStorage.getItem("shopcar")) || []; console.log(shopcarList); this.goodsData.num = 1; this.goodsData.isChecked = false; let ind = shopcarList.findIndex((ele) => { return ele.id == this.goodsId; }); console.log(ind); // 如果有相同的返回该数据的下标,没有则返回-1 if (ind != -1) { shopcarList[ind].num++; } else { shopcarList.push(this.goodsData); } localStorage.setItem("shopcar", JSON.stringify(shopcarList)); this.$router.push("/shopcar"); }, // 立即结算 payFn() { localStorage.setItem("goodspay", JSON.stringify(this.goodsData)); this.$router.push("/pay"); }, }, async created() { this.goodsId = this.$route.query.id; try { let { data } = await getGoodsDetailApi(this.goodsId); this.goodsData = data.data.goodsData; console.log(this.goodsData); } catch (err) { console.log(err); } }, };怎么给页面滚动事件加个背景颜色淡入淡出
你可以在 `data` 中添加一个变量 `searchBgc` 来控制搜索栏背景颜色,然后在 `watch` 中监听 `scrollTop` 的变化,当 `scrollTop` 大于 100 时,将 `searchBgc` 设置为需要的颜色值,同时设置 `opacity` 为 1,实现淡入效果;当 `scrollTop` 小于等于 100 时,将 `searchBgc` 设置为另一个颜色值,同时设置 `opacity` 为 0,实现淡出效果。具体代码如下:
```javascript
export default {
data() {
return {
// 其他变量...
searchBgc: "rgba(0, 0, 0, 0.1)",
opacity: 0
};
},
watch: {
scrollTop: {
handler(newValue, oldValue) {
clearTimeout(this.timer);
this.timer = setTimeout(() => {
if (newValue == window.scrollY) {
this.oldScrollTop = newValue;
if (this.oldScrollTop > 100) {
this.show = true;
this.searchBgc = "rgba(0, 0, 0, 0.6)"; // 设置背景颜色为深色
this.opacity = 1; // 设置透明度为 1,实现淡入效果
} else {
this.show = false;
this.searchBgc = "rgba(0, 0, 0, 0.1)"; // 设置背景颜色为浅色
this.opacity = 0; // 设置透明度为 0,实现淡出效果
}
} else {
if (this.oldScrollTop == oldValue) {
// console.log("滚动开始");
}
}
}, 20);
},
immediate: true
}
},
// 其他方法...
};
```
相关推荐
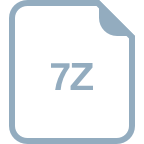
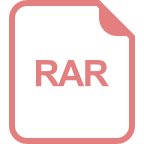
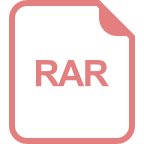












