基于Androidstudio的蓝牙通信客户端代码
时间: 2024-05-11 17:18:37 浏览: 115
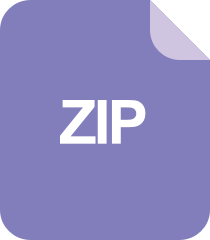
Android Studio的无线传输WIFI工程/TCP客户端版本,支持收、发数据,并包含绘图组件 可供电赛使用
以下是一个基于 Android Studio 的蓝牙通信客户端代码示例:
```java
public class BluetoothActivity extends AppCompatActivity {
private BluetoothAdapter bluetoothAdapter;
private BluetoothDevice device;
private BluetoothSocket socket;
private OutputStream outputStream;
private InputStream inputStream;
private TextView textView;
private final UUID uuid = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_bluetooth);
textView = findViewById(R.id.text_view);
// 获取蓝牙适配器
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
Toast.makeText(this, "设备不支持蓝牙", Toast.LENGTH_SHORT).show();
finish();
return;
}
// 检查蓝牙是否已开启
if (!bluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, 1);
}
// 扫描蓝牙设备
Set<BluetoothDevice> pairedDevices = bluetoothAdapter.getBondedDevices();
if (pairedDevices.size() > 0) {
for (BluetoothDevice d : pairedDevices) {
if (d.getName().equals("设备名称")) {
device = d;
break;
}
}
}
// 连接蓝牙设备
try {
socket = device.createRfcommSocketToServiceRecord(uuid);
socket.connect();
outputStream = socket.getOutputStream();
inputStream = socket.getInputStream();
} catch (IOException e) {
e.printStackTrace();
}
// 读取蓝牙设备发送的数据
new Thread(new Runnable() {
@Override
public void run() {
byte[] buffer = new byte[1024];
int bytes;
while (true) {
try {
bytes = inputStream.read(buffer);
String message = new String(buffer, 0, bytes);
runOnUiThread(new Runnable() {
@Override
public void run() {
textView.setText(message);
}
});
} catch (IOException e) {
e.printStackTrace();
break;
}
}
}
}).start();
}
// 发送数据到蓝牙设备
public void sendData(String data) {
try {
outputStream.write(data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
protected void onDestroy() {
super.onDestroy();
// 关闭蓝牙连接
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上述代码中,`BluetoothActivity` 类继承自 `AppCompatActivity` 类,实现了蓝牙通信的功能。其中,`onCreate` 方法中进行了蓝牙设备的连接和数据读取的操作。`sendData` 方法用于发送数据到蓝牙设备。在 `onDestroy` 方法中,关闭了蓝牙连接。
需要注意的是,该代码示例中的蓝牙设备名称为“设备名称”,需要根据实际情况进行修改。
阅读全文
相关推荐
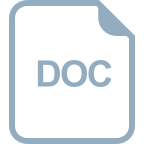
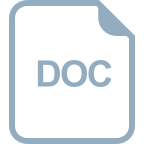

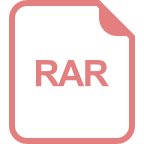
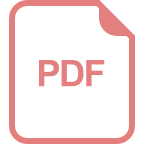
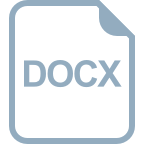
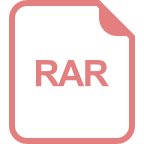
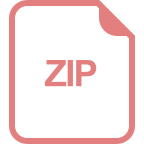
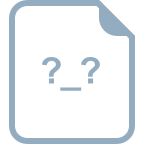
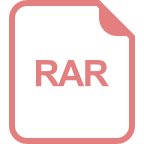
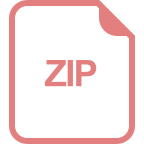
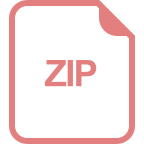
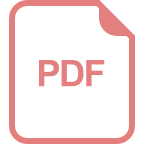
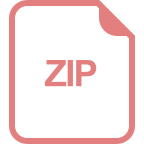
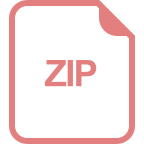
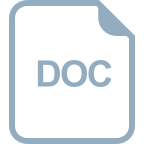
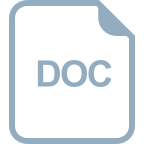
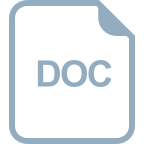