@RequiresPermissions("system:user:edit") @GetMapping("/edit/{userId}") public String edit(@PathVariable("userId") Long userId, ModelMap mmap) { userService.checkUserDataScope(userId); List<SysRole> roles = roleService.selectRolesByUserId(userId); mmap.put("user", userService.selectUserById(userId)); mmap.put("roles", SysUser.isAdmin(userId) ? roles : roles.stream().filter(r -> !r.isAdmin()).collect(Collectors.toList())); mmap.put("posts", postService.selectPostsByUserId(userId)); return prefix + "/edit"; }
时间: 2024-04-26 19:22:11 浏览: 25
这是一个基于Spring框架的Java Web应用中,用户模块的一个Controller类中的一个方法。该方法使用了@GetMapping注解,表示可以通过HTTP GET请求访问该方法。在该方法中,首先通过@PathVariable注解将userId作为路径变量进行获取,然后通过userService中的checkUserDataScope方法,检查当前用户是否具有编辑该用户数据的权限。接着通过roleService中的selectRolesByUserId和postService中的selectPostsByUserId方法,分别获取该用户的角色列表和岗位列表,并将这些数据存入ModelMap中,以便在前端页面中渲染。该方法最后返回了一个字符串,其中prefix是一个字符串变量,表示前缀,用于指定返回的视图名称,这里是“/edit”,表示返回一个名为“edit”的视图。通常在返回的视图中会使用到前面存入的用户信息、角色列表和岗位列表等数据。同时,该方法还使用了@RequiresPermissions注解,表示该方法需要用户具有“system:user:edit”权限才能访问。
相关问题
@RequiresPermissions("system:user:view") @GetMapping("/importTemplate") @ResponseBody public AjaxResult importTemplate() { ExcelUtil<SysUser> util = new ExcelUtil<SysUser>(SysUser.class); return util.importTemplateExcel("用户数据"); }
这是一个基于Spring框架的Java Web应用中,用户模块的一个Controller类中的一个方法。该方法使用了@GetMapping注解,表示可以通过HTTP GET请求访问该方法,同时该方法也使用了@ResponseBody注解,表示该方法的返回值会被直接写入HTTP响应体中。在该方法中,通过ExcelUtil工具类,导出了一个名为“用户数据”的Excel模板,返回给前端。同时,该方法还使用了@RequiresPermissions注解,表示该方法需要用户具有“system:user:view”权限才能访问。
@RequiresPermissions注解 怎么使用
@RequiresPermissions注解是Shiro权限控制框架中的注解,用于控制用户是否有权限执行某个操作或访问某个资源。可以在Controller类或Controller中的方法上使用该注解。
使用方法如下:
1. 在pom.xml文件中添加Shiro依赖:
```
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring</artifactId>
<version>1.4.2</version>
</dependency>
```
2. 在Shiro配置文件中配置安全管理器和权限控制器等相关信息。
3. 在Controller类或Controller中的方法上添加@RequiresPermissions注解,指定权限字符串。
示例代码:
```
@Controller
@RequestMapping("/user")
public class UserController {
@RequiresPermissions("user:list")
@GetMapping("/list")
public String list() {
// 返回用户列表页面
return "user/list";
}
@RequiresPermissions("user:add")
@GetMapping("/add")
public String add() {
// 返回添加用户页面
return "user/add";
}
@RequiresPermissions("user:edit")
@GetMapping("/edit")
public String edit() {
// 返回编辑用户页面
return "user/edit";
}
@RequiresPermissions("user:delete")
@PostMapping("/delete")
@ResponseBody
public String delete() {
// 删除用户
return "success";
}
}
```
在上面的示例代码中,@RequiresPermissions注解用于限制用户访问/user/list、/user/add、/user/edit和/user/delete这些资源的权限。当用户没有相应的权限时,将会抛出UnauthorizedException异常,表示访问被拒绝。
相关推荐
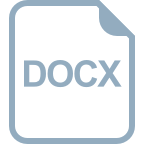
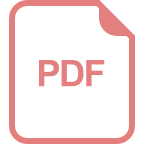
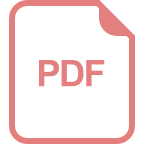













