用keil在stm32f103c8t6实现hc-05蓝牙接收字符串
时间: 2023-09-02 17:06:09 浏览: 438
以下是使用Keil在STM32F103C8T6实现HC-05蓝牙接收字符串的步骤:
1. 在Keil中创建一个新的工程,并选择适当的芯片型号。
2. 在工程文件夹中创建一个新的C文件,命名为main.c。
3. 在main.c文件中添加必要的头文件和宏定义:
```
#include "stm32f10x.h"
#include <stdio.h>
#define USART_TX_PIN GPIO_Pin_9
#define USART_RX_PIN GPIO_Pin_10
#define USART_GPIO GPIOA
#define USART USART1
```
4. 在main函数中初始化USART串口通信:
```
int main(void)
{
// 初始化USART串口通信
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
// 使能USART和GPIO的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART的引脚
GPIO_InitStructure.GPIO_Pin = USART_TX_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(USART_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = USART_RX_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(USART_GPIO, &GPIO_InitStructure);
// 配置USART
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART, &USART_InitStructure);
// 配置USART中断
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART, USART_IT_RXNE, ENABLE);
USART_Cmd(USART, ENABLE);
while (1) {}
}
```
5. 实现USART的中断处理函数:
```
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART, USART_IT_RXNE) != RESET)
{
char ch = USART_ReceiveData(USART);
printf("%c", ch);
}
}
```
6. 在main函数中添加一个无限循环来保持程序运行。
7. 在工程选项中配置串口的调试输出,以便在调试期间查看串口接收到的数据。
8. 将HC-05蓝牙模块连接到STM32F103C8T6开发板的USART1串口引脚。
9. 启动程序并开始与HC-05蓝牙模块进行通信。你应该能够通过Keil的调试输出窗口看到串口接收到的数据。
注意:此示例仅适用于接收字符串,如果你需要发送数据,请使用USART_SendData函数发送数据。
阅读全文
相关推荐
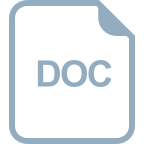
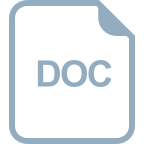
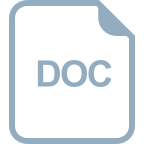

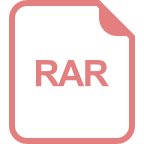
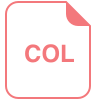
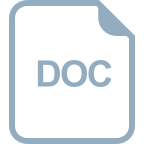
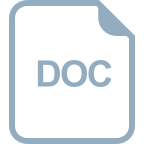
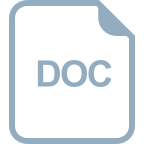
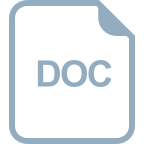
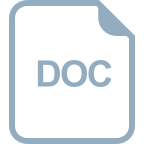
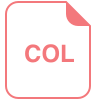


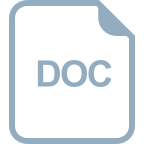
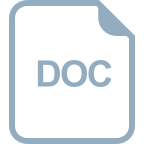
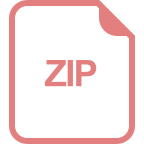
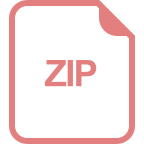
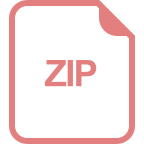