transform::Rigid3f
时间: 2023-09-13 15:03:24 浏览: 35
`transform::Rigid3f` 是Google Cartographer SLAM系统中的一个类,用于表示3D刚体变换。它由一个3D旋转和一个3D平移组成,可以将一个3D点从一个坐标系变换到另一个坐标系。这个类提供了一些方法来进行刚体变换的组合、反转、插值等操作,是Cartographer系统中非常重要的一个组件。
相关问题
transform::Rigid3d
transform::Rigid3d 是 Google Cartographer 库中的一个类,用于表示一个三维刚体变换。它由一个旋转和一个平移组成,可以将一个坐标系中的点映射到另一个坐标系中。其中旋转用四元数表示,平移用三维向量表示。transform::Rigid3d 提供了一些方便的成员函数和操作符,例如乘法、逆变换、插值等。它在机器人定位和地图构建等领域有广泛的应用。
transform::Rigid2d
transform::Rigid2d是一个在ROS(机器人操作系统)中常用的刚体变换类。它用于表示二维空间中的刚体变换,包括平移和旋转。
Rigid2d类提供了以下主要功能:
1. 平移:可以通过x和y坐标来表示平移的向量。
2. 旋转:可以通过角度来表示旋转的量。
3. 刚体变换:可以将平移和旋转组合成一个刚体变换矩阵。
4. 变换操作:可以对点、向量或其他刚体变换进行变换操作。
Rigid2d类的使用可以帮助机器人在二维空间中进行定位、导航和运动规划等任务。它在机器人感知、SLAM(同时定位与地图构建)和路径规划等领域都有广泛的应用。
相关推荐
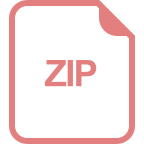
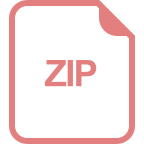












