循环链表约瑟夫环问题并用C语言实现
时间: 2023-05-13 19:06:57 浏览: 139
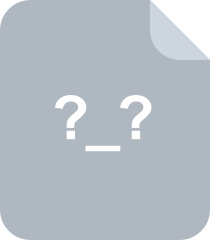
约瑟夫环问题通过循环链表实现(c语言版)
好的,循环链表约瑟夫环问题可以用C语言实现。以下是一个示例代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
Node *createList(int n) {
Node *head = NULL, *tail = NULL;
for (int i = 1; i <= n; i++) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = i;
node->next = NULL;
if (head == NULL) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
tail->next = head;
return head;
}
void josephus(int m, Node **head) {
Node *p = *head, *q = NULL;
while (p->next != p) {
for (int i = 1; i < m; i++) {
q = p;
p = p->next;
}
q->next = p->next;
printf("%d ", p->data);
free(p);
p = q->next;
}
*head = p;
}
int main() {
int n, m;
printf("请输入总人数n和报数m:");
scanf("%d%d", &n, &m);
Node *head = createList(n);
josephus(m, &head);
printf("%d\n", head->data);
return 0;
}
```
这个程序会先创建一个包含n个节点的循环链表,然后按照报数m的规则,依次删除节点,直到只剩下一个节点为止。最后输出剩下的节点的编号。
阅读全文
相关推荐
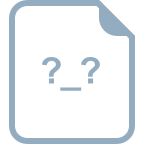
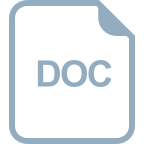
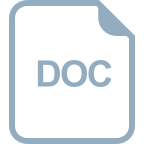
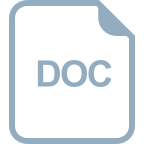
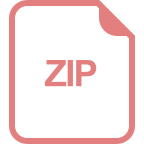
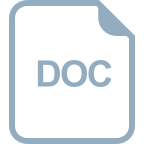
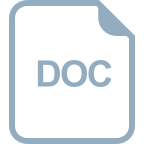
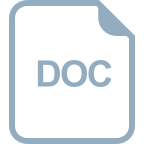
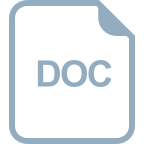
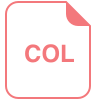




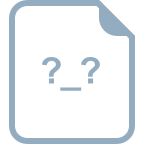
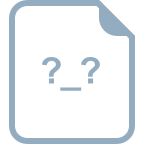
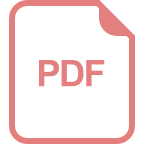