修改这个错误:unsigned char getTemp() { // 这里为了演示方便,我们使用随机数模拟温度传感器的读取 return (unsigned char)(rand() % 51) + 15; // 生成 15 ~ 65 的随机数
时间: 2024-03-15 11:44:10 浏览: 58
这段代码没有明确指定随机数的种子,因此每次运行程序得到的随机数序列都是相同的,可以通过使用time函数来生成一个随机的种子,修改后的代码如下:
```
unsigned char getTemp() {
// 这里为了演示方便,我们使用随机数模拟温度传感器的读取
srand((unsigned)time(NULL)); // 生成随机种子
return (unsigned char)(rand() % 51) + 15; // 生成 15 ~ 65 的随机数
}
```
这样每次程序运行时都会生成不同的随机数序列,提高了代码的随机性。
相关问题
解释这段代码int madplay_state(void)//返回0未在播放 返回1正在播放 { FILE *fp; char buf[10]; memset(buf,'\0',10); fp = popen("ps -ef | grep -w madplay | grep -v grep | wc -l ","r"); fread(buf,1,1,fp);//从给定流 fp 读取数据到 buf 所指向的数组中 pclose(fp); int m = (int)buf[0];//返回值为ASCI码 0对应48 return m-48; } int rand_num(void) { srand((unsigned)time(NULL));//初始化随机数发生器 int a = rand() % (music_num-1); //printf("rand_num:%d\n", a); return a; }
这段代码包含两个函数,分别是 `madplay_state()` 和 `rand_num()`。
`madplay_state()` 函数的作用是检查当前是否在播放音乐。它使用 `popen()` 函数执行 shell 命令 `ps -ef | grep -w madplay | grep -v grep | wc -l`,将结果读入缓冲区 `buf` 中。然后,使用 `pclose()` 函数关闭文件流,并将 `buf[0]` 转成整数,再减去 48(即 `'0'` 的 ASCII 码),得到实际的数字。如果结果为 0,则表示当前未在播放音乐;如果结果为 1,则表示当前正在播放音乐。
`rand_num()` 函数用于随机选取一首歌曲播放。它使用 `srand()` 函数初始化随机数发生器,然后使用 `rand()` 函数生成一个随机数并对歌曲总数取余数,得到一个介于 0(包含)和歌曲总数(不包含)之间的随机数。该随机数就是选取的歌曲的编号,最后将该数字作为函数的返回值。
#include <stdio.h> #include <stdlib.h> #include <time.h> #include <opencv2/opencv.hpp> using namespace cv; int main() { Mat src = imread("C:/Users/iris/Pictures/scenery.jpg"); Mat dst = Mat::zeros(src.size(), src.type()); int rows = src.rows, cols = src.cols; int offsets = 5, random_num = 0; srand((unsigned)time(NULL)); for (int y = 0; y < rows - offsets; ++y) { for (int x = 0; x < cols - offsets; ++x) { random_num = rand() % offsets; dst.at<Vec3b>(y, x)[0] = src.at<Vec3b>(y + random_num, x + random_num)[0]; dst.at<Vec3b>(y, x)[1] = src.at<Vec3b>(y + random_num, x + random_num)[1]; dst.at<Vec3b>(y, x)[2] = src.at<Vec3b>(y + random_num, x + random_num)[2]; } } imshow("src", src); imshow("dst", dst); waitKey(0); destroyAllWindows(); return 0;不用其他库用c语言实现
抱歉,您提供的代码是使用了OpenCV库的C++代码,而您要求使用C语言实现。在C语言中,没有现成的图像处理库,因此需要使用其他第三方库或手动编写图像处理算法来实现。以下是使用C语言手动编写的简单图像处理算法,可以实现对图像进行随机偏移的效果:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define BMP_HEADER_SIZE 54
int main(void)
{
FILE *fp_in, *fp_out;
unsigned char header[BMP_HEADER_SIZE];
int width, height, bit_count, line_size, offset, random_num;
unsigned char *img_in, *img_out;
srand((unsigned)time(NULL));
fp_in = fopen("input.bmp", "rb");
fp_out = fopen("output.bmp", "wb");
if (fp_in == NULL || fp_out == NULL) {
printf("Failed to open files!\n");
return 1;
}
fread(header, sizeof(unsigned char), BMP_HEADER_SIZE, fp_in);
width = *(int *)&header[18];
height = *(int *)&header[22];
bit_count = *(short *)&header[28];
line_size = (bit_count * width + 31) / 32 * 4;
offset = line_size - bit_count / 8 * width;
img_in = (unsigned char *)malloc(sizeof(unsigned char) * height * line_size);
img_out = (unsigned char *)malloc(sizeof(unsigned char) * height * line_size);
fread(img_in, sizeof(unsigned char), height * line_size, fp_in);
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
random_num = rand() % 5;
img_out[y * line_size + x * bit_count / 8 + 0] = img_in[(y + random_num) * line_size + (x + random_num) * bit_count / 8 + 0];
img_out[y * line_size + x * bit_count / 8 + 1] = img_in[(y + random_num) * line_size + (x + random_num) * bit_count / 8 + 1];
img_out[y * line_size + x * bit_count / 8 + 2] = img_in[(y + random_num) * line_size + (x + random_num) * bit_count / 8 + 2];
}
}
fwrite(header, sizeof(unsigned char), BMP_HEADER_SIZE, fp_out);
fwrite(img_out, sizeof(unsigned char), height * line_size, fp_out);
free(img_in);
free(img_out);
fclose(fp_in);
fclose(fp_out);
return 0;
}
```
该代码使用了BMP文件格式,可以将图片保存为BMP格式后进行处理。在代码中,首先读取BMP文件头信息,然后根据图片的宽度、高度和每像素位数计算出每行像素数据的字节数。接着使用随机数生成器生成每个像素的随机偏移量,然后将原图像中的像素进行偏移,输出到新的图像中。最后将新的图像保存为BMP格式。
需要注意的是,该算法仅为简单的示例代码,可能存在一些局限性和缺陷。在实际应用中,需要根据具体的需求和情况进行优化和改进。
阅读全文
相关推荐
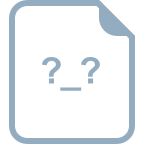
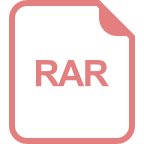
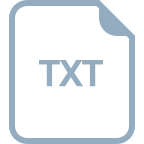













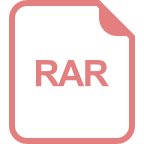
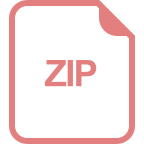