编写一个系统调用,来统计某个特定系统调用被使用的次数
时间: 2023-06-25 16:05:16 浏览: 63
这里给出一个伪代码实现:
1. 定义一个全局变量,用于记录特定系统调用被使用的次数,初始值为 0。
```
int count = 0;
```
2. 在系统调用实现函数中,每次调用特定系统调用时,将该全局变量加 1。
```
// 假设特定系统调用的编号为 123
if (syscall_number == 123) {
count++;
}
```
3. 定义一个新的系统调用,用于查询特定系统调用被使用的次数。
```
long sys_get_count(void) {
return count;
}
```
4. 将该系统调用注册到内核中。
```
// 假设系统调用号为 4242
static const struct file_operations count_fops = {
.owner = THIS_MODULE,
.unlocked_ioctl = sys_get_count
};
static int __init init_syscalls(void) {
int err;
err = register_chrdev(4242, "count", &count_fops);
if (err < 0) {
return err;
}
return 0;
}
static void __exit exit_syscalls(void) {
unregister_chrdev(4242, "count");
}
module_init(init_syscalls);
module_exit(exit_syscalls);
```
5. 用户程序可以通过调用新注册的系统调用来查询特定系统调用被使用的次数。
```
#include <sys/ioctl.h>
#include <stdio.h>
#define GET_COUNT _IOR('c', 1, int)
int main() {
int fd, count;
fd = open("/dev/count", 0);
if (fd < 0) {
perror("open");
return 1;
}
if (ioctl(fd, GET_COUNT, &count) < 0) {
perror("ioctl");
return 1;
}
printf("count = %d\n", count);
close(fd);
return 0;
}
```
注意事项:
1. 实际的实现需要考虑多线程并发访问的问题,可以使用原子操作或锁来保护全局变量。
2. 特定系统调用的编号需要根据实际情况进行调整。
3. 系统调用号需要在内核中唯一,不与其他系统调用冲突。
相关推荐
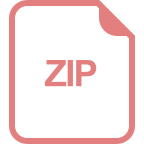
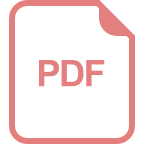
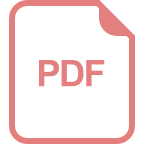














