vue3使用watch
时间: 2023-06-28 17:14:40 浏览: 65
在 Vue 3 中,`watch` 的用法与 Vue 2 中基本相同,但在实现上有些细微的差异。下面是 Vue 3 中使用 `watch` 的基本用法:
1. 监听响应式数据
```
<script>
import { ref, watch } from 'vue';
export default {
setup() {
const count = ref(0);
watch(count, (newValue, oldValue) => {
console.log(`count: ${oldValue} -> ${newValue}`);
});
return {
count,
};
},
};
</script>
```
在上面的代码中,我们使用 `ref` 创建了一个响应式变量 `count`,然后使用 `watch` 监听了这个变量的变化。当 `count` 的值发生改变时,回调函数会被触发,并打印出旧值和新值。
2. 监听多个响应式数据
```
<script>
import { ref, watch } from 'vue';
export default {
setup() {
const count1 = ref(0);
const count2 = ref(0);
watch([count1, count2], ([newCount1, newCount2], [oldCount1, oldCount2]) => {
console.log(`count1: ${oldCount1} -> ${newCount1}`);
console.log(`count2: ${oldCount2} -> ${newCount2}`);
});
return {
count1,
count2,
};
},
};
</script>
```
在上面的代码中,我们使用 `watch` 监听了两个响应式变量 `count1` 和 `count2` 的变化。当这两个变量的值发生改变时,回调函数会被触发,并打印出旧值和新值。
3. 监听非响应式数据
```
<script>
import { ref, watch } from 'vue';
export default {
setup() {
let count = 0;
watch(
() => count,
(newValue, oldValue) => {
console.log(`count: ${oldValue} -> ${newValue}`);
}
);
setInterval(() => {
count++;
}, 1000);
return {};
},
};
</script>
```
在上面的代码中,我们使用 `watch` 监听了一个非响应式变量 `count` 的变化。为了让 `watch` 能够监听到这个变量的变化,我们需要将其包装成一个可以被监听的函数。在这个例子中,我们使用了一个箭头函数来包装 `count`,并在这个函数中返回 `count` 的值。这样,当 `count` 的值发生改变时,回调函数就会被触发,并打印出旧值和新值。
值得注意的是,当我们监听一个非响应式变量时,`watch` 的第一个参数必须是一个函数,不能直接传入变量名。
相关推荐
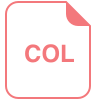
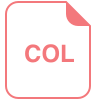
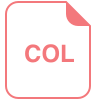
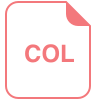
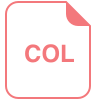









