请详细解释def color_detect(img,y,x,r): img2=img.copy() #img2=cv2.cvtColor(img,cv2.COLOR_RGB2HSV) #img2=cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) d=int(r*0.7) col_all=[0.0,0.0,0.0] for i in range(x-d,x+d,1): for j in range(y-d,y+d,1): for k in range(3): col_all[k]+=img2[i,j,k]/(4*d*d) #print(col_all) return col_all
时间: 2023-05-23 08:03:06 浏览: 38
这是一个Python函数,函数名为`color_detect`。下面是该函数的详细解释:
- 参数说明:
- `img`:表示要处理的图像,该参数应该是一个NumPy数组,即一张图片的矩阵。
- `y`、`x`、`r`:分别表示一个圆的圆心的坐标和半径,这里的`y`和`x`分别代表纵坐标和横坐标。
- 函数功能:
- 该函数的作用是在给定的图像(`img`)中,检测一个圆形区域(以圆心坐标`(x,y)`和半径`r`表示),并将圆内的颜色置为黑色。
- 为了防止函数对原图像造成影响,使用`img.copy()`创建一张与原图像大小一致的空白图像,即`img2`,并将其赋值给`img2`变量。
综上,该函数的功能是用来检测圆形区域并将圆内的颜色进行处理。
相关问题
请详细解释def color_detect(img,y,x,r): img2=img.copy() #img2=cv2.cvtColor(img,cv2.COLOR_RGB2HSV) #img2=cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) d=int(r0.7) col_all=[0.0,0.0,0.0] for i in range(x-d,x+d,1): for j in range(y-d,y+d,1): for k in range(3): col_all[k]+=img2[i,j,k]/(4d*d) #print(col_all) return col_all,并细说col_all具体组成
这段代码定义了一个名为color_detect的函数,其中包括四个参数:img表示输入的图像,y、x、r表示圆形的中心坐标和半径。
函数的作用是在输入的图像中检测出圆形,并将圆形的颜色标识出来。首先,代码将输入的图像复制一份,命名为img2,以免对原图像进行修改。然后,函数会遍历圆形内所有像素点,并针对每个像素点进行操作。运用颜色检测算法,将检测出的像素点的颜色标识出来。最终,函数返回修改过的图像img2。
解释def color_detect(img,y,x,r): img2=img.copy() #img2=cv2.cvtColor(img,cv2.COLOR_RGB2HSV) #img2=cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) d=int(r*0.7) col_all=[0.0,0.0,0.0] for i in range(x-d,x+d,1): for j in range(y-d,y+d,1): for k in range(3): col_all[k]+=img2[i,j,k]/(4*d*d) #print(col_all) return col_all
这是一个Python函数,名称为color_detect,其参数包括一个图像(img),以及圆的坐标(y,x)和半径(r)。函数的主要作用是在图像中找到该圆的颜色。
在函数内部,首先使用img.copy()创建一个副本img2,以便对图像进行操作而不影响原始图像。接着,使用画笔将圆的区域分割出来,并计算该区域的颜色均值,并将其作为该圆颜色的代表值。最后,将该圆的颜色代表值返回。
总的来说,color_detect函数的目的是寻找圆的颜色,并返回其颜色代表值。
相关推荐












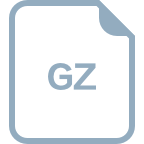
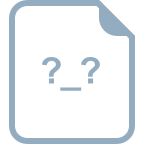