recyclerview设置背景 并且头尾圆角
时间: 2023-08-19 13:05:52 浏览: 134
要给 RecyclerView 设置背景并且设置头部和尾部圆角,可以采用以下步骤:
1. 定义一个自定义的 Drawable,可以在 drawable 目录下新建一个 XML 文件,命名为 round_bg.xml,代码如下:
```
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<corners android:topLeftRadius="10dp"
android:topRightRadius="10dp"
android:bottomLeftRadius="10dp"
android:bottomRightRadius="10dp"/>
<solid android:color="@color/white"/>
</shape>
```
该 Drawable 定义了一个矩形的圆角背景,四个角的圆角半径均为 10dp,填充颜色为白色。
2. 在 RecyclerView 的布局文件中,设置背景为该 Drawable。例如:
```
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/round_bg"/>
```
3. 在 RecyclerView 的 Adapter 中,重写 getItemViewType 方法,在其中判断当前位置是否为头部或尾部,返回不同的 ViewType。例如:
```
@Override
public int getItemViewType(int position) {
if (position == 0) {
return VIEW_TYPE_HEADER;
} else if (position == getItemCount() - 1) {
return VIEW_TYPE_FOOTER;
} else {
return VIEW_TYPE_ITEM;
}
}
```
4. 在 onCreateViewHolder 方法中创建对应的 ViewHolder,并根据 ViewType 设置不同的布局和背景。例如:
```
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
if (viewType == VIEW_TYPE_HEADER) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.header_layout, parent, false);
return new HeaderViewHolder(view);
} else if (viewType == VIEW_TYPE_FOOTER) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.footer_layout, parent, false);
return new FooterViewHolder(view);
} else {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.item_layout, parent, false);
return new ItemViewHolder(view);
}
}
```
5. 最后,在对应的布局文件中,可以根据需求自定义头部和尾部的样式,并设置圆角。例如:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/round_bg"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/header_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Header"
android:textColor="@color/black"
android:textSize="16sp" />
</LinearLayout>
```
以上就是在 RecyclerView 中设置背景并且设置头部和尾部圆角的步骤。
阅读全文
相关推荐
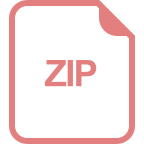


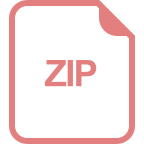
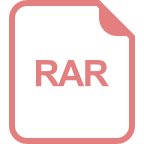
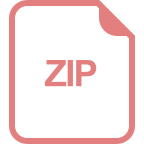
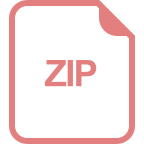
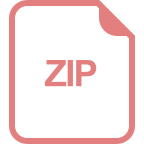
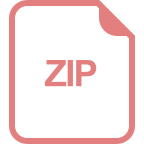
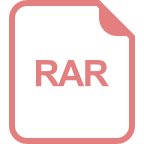
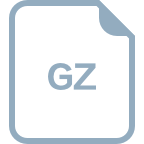
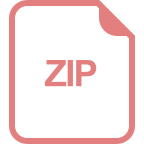
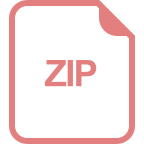
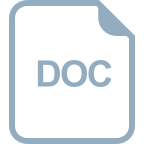
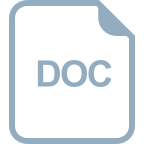
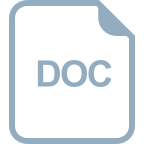