ValueError: x and y must have same first dimension, but have shapes (1,) and (1000,)
时间: 2023-11-29 18:06:18 浏览: 55
这个错误是由于 x 和 y 的长度不一致导致的。你需要确保 x 和 y 的长度相同。你可以尝试使用 `np.arange()` 函数来生成一个与 y 相同长度的数组,或者重新定义 x 以匹配 y 的长度。以下是两种方法的示例代码:
方法一:使用 `np.arange()` 函数生成一个与 y 相同长度的数组
```
import numpy as np
import matplotlib.pyplot as plt
y = np.random.randn(1000)
x = np.arange(len(y))
plt.figure()
plt.plot(x, y)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("随机波形")
plt.show()
```
方法二:重新定义 x 以匹配 y 的长度
```
import numpy as np
import matplotlib.pyplot as plt
y = np.random.randn(1000)
x = np.linspace(0, 999, 1000)
plt.figure()
plt.plot(x, y)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("随机波形")
plt.show()
```
这两种方法都可以确保 x 和 y 的长度相同,从而避免了出现该错误。
相关问题
ValueError: x and y must have same first dimension, but have shapes (2000,) and (1,)
`ValueError: x and y must have same first dimension, but have shapes (2000,) and (1,)` 错误通常出现在使用Matplotlib绘制图形时,x轴和y轴的数据长度不一致的情况下。
这个错误提示意味着x轴数据和y轴数据的长度不相等。在这个例子中,x轴数据的长度是2000,而y轴数据的长度是1。这个错误通常发生在以下情况:
1. 没有正确地指定x轴和y轴数据。在使用Matplotlib绘制图形时,需要明确指定x轴和y轴的数据。如果没有正确地指定x轴和y轴数据,可能会导致数据长度不匹配的错误。在绘图函数中,x轴数据通常作为第一个参数传入,而y轴数据通常作为第二个参数传入。
2. x轴和y轴数据长度不匹配。在某些情况下,x轴和y轴数据长度不匹配可能是由于代码逻辑错误或数据处理错误导致的。在这种情况下,需要检查代码逻辑和数据处理过程,并确保x轴和y轴数据长度匹配。
3. 数据类型不匹配。在某些情况下,x轴和y轴数据的数据类型不匹配可能会导致数据长度不匹配的错误。在这种情况下,需要检查数据类型,并确保它们匹配。
以下是一个示例代码,展示如何解决这个错误:
```
import matplotlib.pyplot as plt
# 生成x轴数据
x = range(2000)
# 生成y轴数据
y = [i**2 for i in x]
# 绘制图形
plt.plot(x, y)
plt.xlabel('x')
plt.ylabel('y')
plt.title('Example Plot')
# 显示图形
plt.show()
```
在这个示例代码中,我们生成了一个包含2000个元素的x轴数据,以及一个与x轴数据长度相同的y轴数据。然后,我们使用 `plt.plot()` 函数将这些数据绘制成一条曲线,并添加x轴标签、y轴标签和图形标题。最后,我们使用 `plt.show()` 函数显示图形。通过这个示例代码,我们可以确保x轴和y轴数据长度匹配,并正确地显示图形。
ValueError: x and y must have same first dimension, but have shapes (1000,) and (1,)
This error occurs when you are trying to plot two arrays with different first dimensions. The first dimension of an array refers to its size along the first axis. In this case, the error message indicates that the first dimensions of the two arrays are not the same.
For example, if you are trying to plot a line graph using the Matplotlib library and your x-axis has 1000 values and your y-axis has only one value, this error will occur because there is no way to match up the x and y values.
To fix this error, you need to make sure that the first dimensions of both arrays are the same. This can be done by either changing the shape of the arrays or by selecting a subset of the arrays.
For example, if you have two arrays x and y, and you want to plot them using Matplotlib, you can use the following code:
```python
import matplotlib.pyplot as plt
x = [1,2,3,4,5,6,7,8,9,10]
y = [5,3,6,7,8,5,6,7,8,9]
plt.plot(x, y)
plt.show()
```
In this example, both x and y have the same first dimension (10), so there will be no error when plotting the graph.
相关推荐
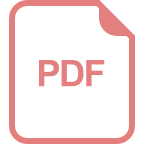
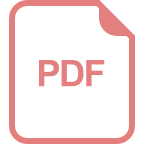
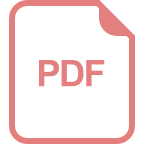












