ValueError: x and y must have same first dimension, but have shapes (89,) and (87, 1)
时间: 2024-05-08 10:17:15 浏览: 26
This error occurs when you are trying to plot a graph with two arrays of different sizes. In this case, the x array has 89 elements and the y array has 87 elements.
To fix this error, you need to make sure that the x and y arrays have the same number of elements. One possible solution is to modify the y array by adding or removing some elements to match the size of the x array.
Another solution is to reshape the y array so that it has the same number of rows as the x array. You can do this by using the numpy.reshape() function as follows:
```
import numpy as np
x = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89])
y = np.array([3.2, 4.5, 5.7, 6.8, 7.9, 9.0, 10.1, 11.2, 12.3, 13.4, 14.5, 15.6, 16.7, 17.8, 18.9, 19.0, 20.1, 21.2, 22.3, 23.4, 24.5, 25.6, 26.7, 27.8, 28.9, 30.0, 31.1, 32.2, 33.3, 34.4, 35.5, 36.6, 37.7, 38.8, 39.9, 41.0, 42.1, 43.2, 44.3, 45.4, 46.5, 47.6, 48.7, 49.8, 50.9, 52.0, 53.1, 54.2, 55.3, 56.4, 57.5, 58.6, 59.7, 60.8, 61.9, 63.0, 64.1, 65.2, 66.3, 67.4, 68.5, 69.6, 70.7, 71.8, 72.9, 74.0, 75.1, 76.2, 77.3, 78.4, 79.5, 80.6, 81.7, 82.8, 83.9, 85.0, 86.1, 87.2, 88.3, 89.4, 90.5, 91.6, 92.7, 93.8, 94.9, 96.0])
# Reshape y array to have the same number of rows as x array
y = y.reshape(-1, 1)
# Plot the graph
import matplotlib.pyplot as plt
plt.plot(x, y)
plt.show()
```
This code will reshape the y array to have 89 rows and 1 column, so that it has the same number of elements as the x array. The graph will then be plotted without any errors.
相关推荐
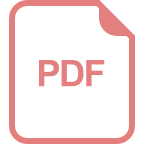
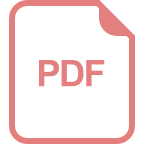
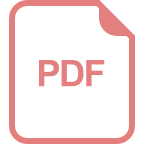














