定义一个包含图书信息(书号、书名、价格)的单链表,读入相应的图书数据来完成图书信息表的创建。然后,统计图书表中的图书个数,同时逐行输出每本书的信息。
时间: 2023-12-23 14:06:27 浏览: 32
以下是Python实现:
```python
class Book:
def __init__(self, book_id, book_name, price):
self.book_id = book_id
self.book_name = book_name
self.price = price
class Node:
def __init__(self, data):
self.data = data
self.next = None
class BookList:
def __init__(self):
self.head = None
self.count = 0
def add_book(self, book):
new_node = Node(book)
if self.head is None:
self.head = new_node
else:
current_node = self.head
while current_node.next is not None:
current_node = current_node.next
current_node.next = new_node
self.count += 1
def print_list(self):
current_node = self.head
while current_node is not None:
print("Book ID: ", current_node.data.book_id)
print("Book Name: ", current_node.data.book_name)
print("Price: ", current_node.data.price)
current_node = current_node.next
book_list = BookList()
while True:
book_id = input("Enter book ID (0 to exit): ")
if book_id == "0":
break
book_name = input("Enter book name: ")
price = float(input("Enter price: "))
book = Book(book_id, book_name, price)
book_list.add_book(book)
print("Total number of books: ", book_list.count)
book_list.print_list()
```
运行程序后,输入图书信息,以0结束输入。程序将逐行输出每本书的信息,同时统计图书总数。
相关推荐
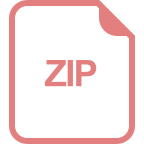
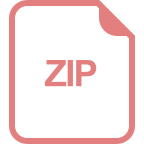














