transforms.compose
时间: 2023-08-25 14:13:19 浏览: 51
`transforms.Compose` 是一个 torchvision 中的函数,用于将多个图像变换组合成一个组合变换。它接受一个变换列表并返回一个新的变换,该变换按照给定的顺序应用每个变换。
例如,以下代码将图像大小调整为 224x224 像素,然后将其转换为 PyTorch 张量:
```
from torchvision import transforms
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor()
])
image = transform(image)
```
在这个例子中,先将图像大小调整为 (224, 224),然后将其转换为 PyTorch 张量。由于 `transforms.Resize` 和 `transforms.ToTensor` 都是变换函数,因此需要使用 `transforms.Compose` 将它们组合在一起。
相关问题
transforms.Compose
transforms.Compose是PyTorch中的一个类,用于将多个图像变换操作组合在一起。它接受一个包含多个变换操作的列表,并按顺序应用这些操作。
例如,假设我们有两个变换操作:Resize和ToTensor。我们可以使用transforms.Compose将它们组合在一起,使得我们可以在应用到图像上时一次性执行这两个操作。
以下是一个使用transforms.Compose的示例代码:
```python
import torch
from torchvision import transforms
# 定义两个变换操作
transform_resize = transforms.Resize((256, 256))
transform_to_tensor = transforms.ToTensor()
# 创建Compose对象并将变换操作组合在一起
composed_transform = transforms.Compose([transform_resize, transform_to_tensor])
# 应用组合的变换操作到图像上
image = torch.randn(3, 300, 300) # 随机生成一个形状为(3, 300, 300)的图像
transformed_image = composed_transform(image)
```
在上面的示例中,我们首先定义了两个变换操作:Resize和ToTensor。然后,我们使用transforms.Compose创建了一个Compose对象,并将这两个变换操作组合在一起。最后,我们将这个组合的变换操作应用到一个随机生成的图像上,得到一个经过变换的图像。
transforms.Compose函数
transforms.Compose函数是PyTorch中的一个类,用于将多个图像预处理操作组合在一起。它接受一个操作列表作为参数,并按照列表中的顺序依次应用这些操作。每个操作都是transforms模块中的一个函数,比如transforms.CenterCrop和transforms.ToTensor等。通过使用transforms.Compose函数,可以方便地将多个预处理操作应用到图像上,以便进行数据增强或数据转换等操作。\[1\]\[2\]\[3\]
#### 引用[.reference_title]
- *1* [不懂pytorch里transforms.Compose()函数的同学看过来!](https://blog.csdn.net/qq_43722906/article/details/115761415)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [Pytorch中transforms.Compose()的使用](https://blog.csdn.net/qq_33287871/article/details/115077178)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
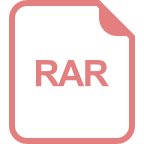
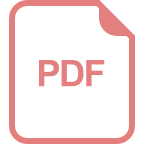
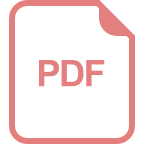



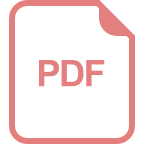
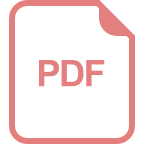
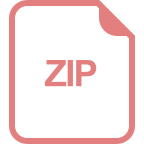
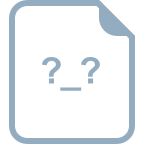
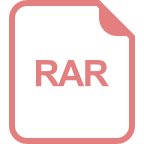
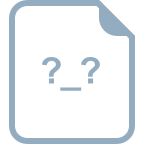
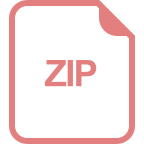
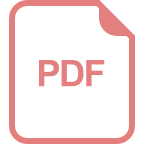