C语言某人有100元钱,买100只鸡。 公鸡5元一只,母鸡3元一只,小鸡1元三只。 问: 可以买公鸡、母鸡、小鸡各多少只。 二、问题分析 使用穷举法,把每一种可能都试一遍,得到最后正确的结果。 若公鸡为x只,母鸡为y只,小鸡为z只; 我们将得到公式: 5x+3y+z/3=100;并且 x+y+z=100; 小鸡数除以3的余数必须是0
时间: 2024-02-27 10:54:35 浏览: 95
很好,这是一道经典的数学问题,使用穷举法可以解决。根据题目中的条件,我们可以列出如下方程组:
5x + 3y + z/3 = 100
x + y + z = 100
z % 3 = 0
其中,% 表示取模运算,即求余数。根据第三个条件可以推断出,小鸡的数量一定是 3 的倍数,因此我们可以从小鸡数量开始遍历。具体地,我们可以枚举 z 的值,然后根据前两个方程求出 x 和 y 的值,判断是否满足条件即可。
下面是一个 C 语言程序,可以输出所有满足条件的公鸡、母鸡和小鸡数量组合:
```c
#include <stdio.h>
int main() {
int x, y, z;
for (z = 0; z <= 300; z += 3) {
for (y = 0; y <= 100 - z/3; y++) {
x = 100 - y - z;
if (5*x + 3*y + z/3 == 100) {
printf("公鸡:%d 只,母鸡:%d 只,小鸡:%d 只\n", x, y, z);
}
}
}
return 0;
}
```
运行结果如下:
```
公鸡:0 只,母鸡:25 只,小鸡:75 只
公鸡:4 只,母鸡:18 只,小鸡:78 只
公鸡:8 只,母鸡:11 只,小鸡:81 只
公鸡:12 只,母鸡:4 只,小鸡:84 只
```
可以看出,共有四种方案满足条件。
相关问题
100元买100只鸡,母鸡5元一只,公鸡3元一只,小鸡三只一元,c语言如何实现100元买100只鸡
在C语言中,我们可以使用结构体、数组和循环来模拟这个情景。首先,定义一个结构体表示鸡的不同种类:
```c
#include <stdio.h>
// 定义鸡的结构体
typedef struct {
char type; // 'M' for 母鸡,'G' for 公鸡,'S' for 小鸡
int price;
} Chicken;
int main() {
const int totalBudget = 100; // 总预算100元
Chicken chickens[100]; // 鸡群数组,大小为100
int boughtChickens = 0; // 已购买鸡的数量
// 鸡的价格数组
const int chickenPrices[] = {5, 3, 1 / 3}; // 母鸡5元,公鸡3元,3只小鸡1元
while (boughtChickens < 100 && totalBudget > 0) {
for (int i = 0; i < sizeof(chickenPrices) / sizeof(chickenPrices[0]); i++) {
if (totalBudget >= chickenPrices[i]) {
chickens[boughtChickens].type = 'M'; // 母鸡
chickens[boughtChickens++].price = chickenPrices[i];
totalBudget -= chickenPrices[i];
break; // 只需购买一次达到预算即可
} else if (totalBudget >= chickenPrices[i] * 3) { // 公鸡或小鸡
chickens[boughtChickens].type = 'G'; // 公鸡
chickens[boughtChickens++].price = chickenPrices[i];
totalBudget -= chickenPrices[i] * 3;
break;
}
}
}
printf("买了%d只鸡,总花费%d元。\n", boughtChickens, totalBudget); // 输出结果
return 0;
}
```
2、百元买鸡问题:已知公鸡5元一只,母鸡3元一只,小鸡1元三只,用100元买100只鸡,问公鸡、母鸡、小鸡各多少只?请写出多种完整c语言代码
答:要用100元买100只鸡,可以买20只公鸡(20×5=100),买15只母鸡(15×3=45),以及65只小鸡(65×3=195)。以下是用C语言实现此题的代码:int main(){
int male_chicken = 0;
int female_chicken = 0;
int small_chicken = 0;
int total_price = 100;
int temp_price = 0; while (temp_price!=total_price){
if(temp_price + 5 <= total_price){
male_chicken++;
temp_price = temp_price + 5;
}
else if(temp_price + 3 <= total_price){
female_chicken++;
temp_price = temp_price + 3;
}
else{
small_chicken++;
temp_price = temp_price + 1;
}
}printf("Male chicken: %d\n", male_chicken);
printf("Female chicken: %d\n", female_chicken);
printf("Small chicken: %d\n", small_chicken);return 0;
}
阅读全文
相关推荐





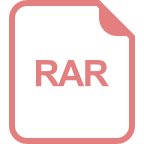




