java 读取word书签_Java 添加、读取、删除Word书签
时间: 2024-01-25 08:02:50 浏览: 188
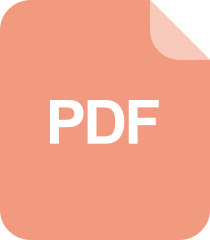
java操作word可操作书签实用.pdf
您可以使用Apache POI库来读取、添加、删除Word文档中的书签。下面是一个简单的示例代码,用于读取文档中的书签:
```java
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.openxml4j.exceptions.InvalidFormatException;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
public class ReadBookmarks {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("sample.docx");
XWPFDocument document = new XWPFDocument(fis);
// Get all paragraphs in the document
List<XWPFParagraph> paragraphs = document.getParagraphs();
// Loop through all paragraphs
for (XWPFParagraph paragraph : paragraphs) {
// Get all runs in the paragraph
List<XWPFRun> runs = paragraph.getRuns();
// Loop through all runs
for (XWPFRun run : runs) {
// Check if the run contains a bookmark
if (run.getCTR().getBookmarkStartList() != null) {
// Loop through all bookmarks in the run
for (CTBookmark bookmark : run.getCTR().getBookmarkStartList()) {
// Print the name of the bookmark
System.out.println("Bookmark: " + bookmark.getName());
}
}
}
}
// Close the document
document.close();
fis.close();
} catch (IOException | InvalidFormatException ex) {
ex.printStackTrace();
}
}
}
```
这个代码将打开名为“sample.docx”的Word文档,并遍历文档中的所有段落和运行。如果运行包含书签,则它将打印书签的名称。
要添加书签,您可以使用以下代码:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.openxml4j.exceptions.InvalidFormatException;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
public class AddBookmark {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("sample.docx");
XWPFDocument document = new XWPFDocument(fis);
// Get the first paragraph in the document
XWPFParagraph paragraph = document.getParagraphs().get(0);
// Create a new run in the paragraph
XWPFRun run = paragraph.createRun();
// Add text to the run
run.setText("This is a bookmark");
// Add a bookmark to the run
run.getCTR().addNewBookmarkStart().setName("bookmark1");
run.getCTR().addNewBookmarkEnd();
// Save the document
FileOutputStream fos = new FileOutputStream("sample.docx");
document.write(fos);
// Close the document
document.close();
fis.close();
fos.close();
} catch (IOException | InvalidFormatException ex) {
ex.printStackTrace();
}
}
}
```
这个代码将在文档的第一个段落中创建一个新的运行,并将一个名为“bookmark1”的书签添加到该运行中。
要删除书签,您可以使用以下代码:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.openxml4j.exceptions.InvalidFormatException;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
public class DeleteBookmark {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("sample.docx");
XWPFDocument document = new XWPFDocument(fis);
// Get the first paragraph in the document
XWPFParagraph paragraph = document.getParagraphs().get(0);
// Loop through all runs in the paragraph
for (XWPFRun run : paragraph.getRuns()) {
// Check if the run contains a bookmark
if (run.getCTR().getBookmarkStartList() != null) {
// Loop through all bookmarks in the run
for (CTBookmark bookmark : run.getCTR().getBookmarkStartList()) {
// Check if the bookmark has the name "bookmark1"
if (bookmark.getName().equals("bookmark1")) {
// Remove the bookmark from the run
run.getCTR().removeBookmark(bookmark);
}
}
}
}
// Save the document
FileOutputStream fos = new FileOutputStream("sample.docx");
document.write(fos);
// Close the document
document.close();
fis.close();
fos.close();
} catch (IOException | InvalidFormatException ex) {
ex.printStackTrace();
}
}
}
```
这个代码将遍历文档的第一个段落中的所有运行,并删除名称为“bookmark1”的书签。
阅读全文
相关推荐


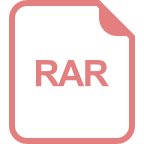
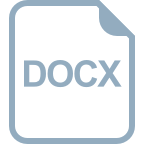
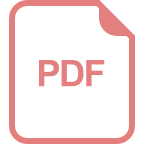
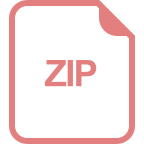
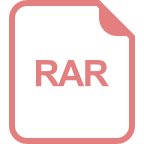
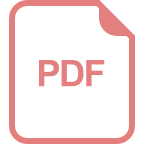
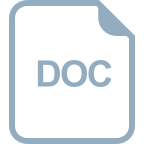
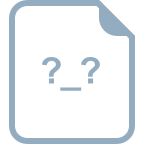
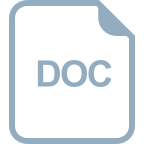



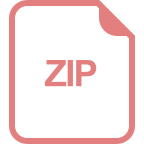