python实现Gentle AdaBoost
时间: 2023-06-29 15:10:52 浏览: 137
Gentle AdaBoost是AdaBoost的一种变体,它通过对弱分类器的输出进行平滑处理来减少分类误差的波动。下面是一个使用Python实现Gentle AdaBoost的例子:
首先,我们需要导入必要的库:numpy和sklearn中的DecisionTreeClassifier。
```python
import numpy as np
from sklearn.tree import DecisionTreeClassifier
```
接下来,我们定义一个Gentle AdaBoost类。在初始化函数中,我们定义了弱分类器的数量、学习率和平滑参数。
```python
class GentleAdaBoost:
def __init__(self, n_estimators=50, learning_rate=1.0, smooth_param=0.1):
self.n_estimators = n_estimators
self.learning_rate = learning_rate
self.smooth_param = smooth_param
self.estimators_ = []
def fit(self, X, y):
weights = np.ones(len(X)) / len(X)
self.estimators_ = []
for _ in range(self.n_estimators):
tree = DecisionTreeClassifier(max_depth=1)
tree.fit(X, y, sample_weight=weights)
predictions = tree.predict(X)
error = np.sum(weights * (predictions != y))
alpha = self.learning_rate * (
np.log((1.0 - error + self.smooth_param) / (error + self.smooth_param)) + np.log(2.0))
weights *= np.exp(alpha * (predictions != y))
weights /= np.sum(weights)
self.estimators_.append((alpha, tree))
def predict(self, X):
n_classes = len(np.unique(y))
res = np.zeros((len(X), n_classes))
for alpha, tree in self.estimators_:
res += alpha * tree.predict_proba(X)
return np.argmax(res, axis=1)
```
在fit函数中,我们首先初始化每个样本的权重为1/n,其中n是样本数量。然后,我们开始迭代,训练每个弱分类器并用它来进行预测。计算错误率和alpha值,更新样本权重,并将弱分类器及其对应的alpha值添加到estimators_列表中。
在predict函数中,我们使用每个弱分类器的预测结果和它们的alpha值来计算加权和,并返回最终的预测结果。
现在,我们可以使用这个Gentle AdaBoost类来训练和预测数据。下面是一个使用鸢尾花数据集的例子:
```python
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2)
model = GentleAdaBoost(n_estimators=50, learning_rate=0.1, smooth_param=0.1)
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
print("Accuracy:", accuracy_score(y_test, y_pred))
```
在这个例子中,我们使用50个弱分类器、学习率为0.1和平滑参数为0.1来训练模型。最后,我们使用测试集来评估模型的准确性。
阅读全文
相关推荐
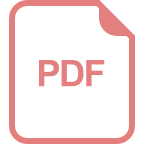
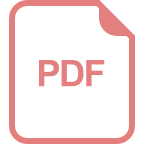
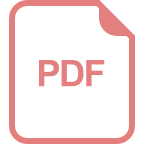
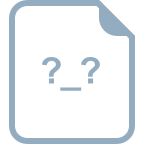
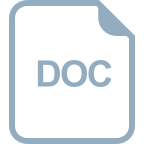
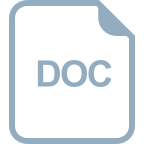

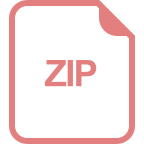
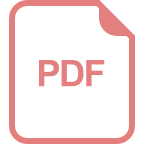
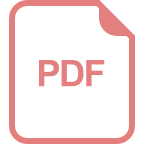
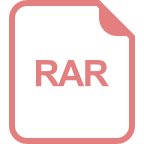
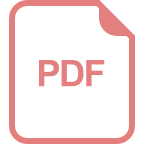
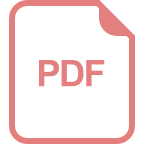
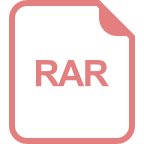
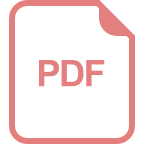
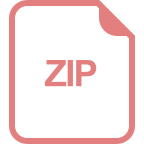