用python实现带权重的BCELoss
时间: 2023-09-19 16:08:24 浏览: 55
以下是用Python实现带权重的BCELoss的示例代码:
```python
import torch.nn.functional as F
import torch
class WeightedBCELoss(torch.nn.Module):
def __init__(self, weight=None):
super(WeightedBCELoss, self).__init__()
self.weight = weight
def forward(self, input, target):
if self.weight is not None:
# apply weights to both the input and target tensors
input = input * self.weight
target = target * self.weight
# apply binary cross entropy loss
loss = F.binary_cross_entropy(input, target, reduction='mean')
return loss
```
该类继承自`torch.nn.Module`,并重写了`forward`方法来计算带权重的BCELoss。如果没有指定权重,则默认为None。
在`forward`方法中,首先检查`weight`是否为None,如果不是,则将权重应用于输入和目标张量。之后,使用Pytorch中的`F.binary_cross_entropy`函数计算二元交叉熵损失。最后,返回计算出的损失。
使用示例:
```python
import torch
# create input and target tensors
input = torch.randn(3, requires_grad=True)
target = torch.tensor([0, 1, 0], dtype=torch.float)
# create weight tensor
weight = torch.tensor([0.5, 1.5, 0.5], dtype=torch.float)
# create instance of WeightedBCELoss class
criterion = WeightedBCELoss(weight=weight)
# compute loss
loss = criterion(input, target)
# print loss
print(loss)
```
在此示例中,输入张量的形状为(3,),目标张量的形状为(3,)。然后,创建一个权重张量,其形状与输入和目标张量的形状相同。最后,创建一个`WeightedBCELoss`类的实例,并使用输入张量和目标张量计算损失。输出为一个标量张量,表示计算出的损失。
相关推荐
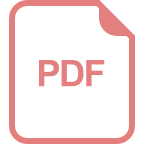
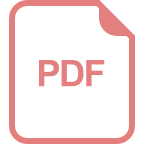
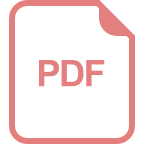














