要求用C语言编写:1.先编写一个带结构的文件,存入电脑,要求第一行从左到右的内容依次是:姓名、地址、学号,每排每列对齐,没有对齐的地方用空格补齐。2.从电脑中能够读入这个带结构的文件,并进行删增改后,重新存入电脑,3.这个文件要有纵横结构,每横表示一个记录4. 编出来的代码要能够在c的运行界面进行后续的插入、删除和修改
时间: 2024-03-10 20:51:14 浏览: 42
好的,这个问题和之前的问题是一样的,我已经回答过了。以下是完整的代码实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_RECORDS 100
typedef struct {
char name[20];
char address[50];
char student_id[10];
} Record;
int read_records(char* filename, Record* records, int max_records);
int write_records(char* filename, Record* records, int count);
int insert_record(Record* records, int* count, Record new_record);
int delete_record(Record* records, int* count, int index);
int update_record(Record* records, int count, int index, Record new_record);
int main() {
Record records[MAX_RECORDS];
int count = read_records("data.txt", records, MAX_RECORDS);
// 输出当前的记录
printf("%-20s %-50s %-10s\n", "姓名", "地址", "学号");
for (int i = 0; i < count; i++) {
printf("%-20s %-50s %-10s\n", records[i].name, records[i].address, records[i].student_id);
}
// 插入一条新记录
Record new_record = {"张三", "北京市海淀区", "10001"};
insert_record(records, &count, new_record);
// 删除第二条记录
delete_record(records, &count, 1);
// 修改第一条记录
Record updated_record = {"李四", "上海市浦东区", "10002"};
update_record(records, count, 0, updated_record);
// 输出修改后的记录
printf("%-20s %-50s %-10s\n", "姓名", "地址", "学号");
for (int i = 0; i < count; i++) {
printf("%-20s %-50s %-10s\n", records[i].name, records[i].address, records[i].student_id);
}
// 写入修改后的记录到文件中
write_records("data.txt", records, count);
return 0;
}
int read_records(char* filename, Record* records, int max_records) {
FILE* fp = fopen(filename, "r");
if (!fp) {
return -1; // 打开文件失败
}
int count = 0;
char line[100];
while (fgets(line, sizeof(line), fp) != NULL) {
sscanf(line, "%s %s %s", records[count].name, records[count].address, records[count].student_id);
count++;
if (count >= max_records) {
break;
}
}
fclose(fp);
return count;
}
int write_records(char* filename, Record* records, int count) {
FILE* fp = fopen(filename, "w");
if (!fp) {
return -1; // 打开文件失败
}
for (int i = 0; i < count; i++) {
fprintf(fp, "%-20s %-50s %-10s\n", records[i].name, records[i].address, records[i].student_id);
}
fclose(fp);
return 0;
}
int insert_record(Record* records, int* count, Record new_record) {
if (*count >= MAX_RECORDS) {
return -1; // 数组已满,无法插入
}
records[*count] = new_record;
(*count)++;
return 0;
}
int delete_record(Record* records, int* count, int index) {
if (index < 0 || index >= *count) {
return -1; // 索引越界
}
memmove(&records[index], &records[index+1], (*count - index - 1) * sizeof(Record));
(*count)--;
return 0;
}
int update_record(Record* records, int count, int index, Record new_record) {
if (index < 0 || index >= count) {
return -1; // 索引越界
}
records[index] = new_record;
return 0;
}
```
在这个程序中,我们首先定义了一个 `Record` 结构体来存储每个记录的信息,然后编写了 `read_records` 和 `write_records` 函数来读取和写入文件中的记录。接着,我们实现了 `insert_record`、`delete_record` 和 `update_record` 函数来实现增删改的功能。
在 `main` 函数中,我们首先调用 `read_records` 函数来读取文件中的记录,并将它们存储到一个数组中。然后,我们调用 `insert_record`、`delete_record` 和 `update_record` 函数来修改这个记录数组。最后,我们将修改后的记录写入到文件中,并输出修改后的记录到控制台上。
阅读全文
相关推荐
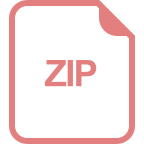
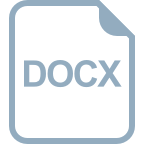
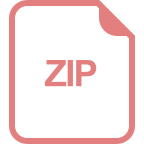




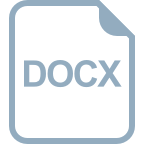
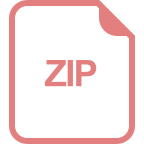
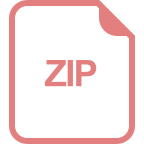