boost实现FTP文件下载
时间: 2024-06-08 11:12:31 浏览: 157
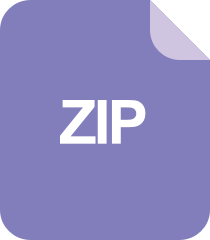
用C++实现FTP的上传下载功能,对网络网络编程感兴趣的朋友可能会用到.zip

Boost库提供了asio网络编程库,可以用它来实现FTP文件下载。以下是一个简单的示例代码,可以下载FTP服务器上的文件:
```c++
#include <iostream>
#include <fstream>
#include <boost/asio.hpp>
#include <boost/filesystem.hpp>
using namespace boost::asio;
using namespace boost::asio::ip;
using namespace boost::filesystem;
class ftp_client {
public:
ftp_client(io_service& ios) : m_sock(ios) {}
void download(const std::string& url, const std::string& user, const std::string& password, const std::string& remote_path, const std::string& local_path) {
// 解析URL
std::string host;
uint16_t port = 21;
std::string path;
if (!parse_url(url, host, port, path)) {
std::cerr << "Invalid URL: " << url << std::endl;
return;
}
// 连接FTP服务器
tcp::resolver resolver(m_sock.get_io_service());
tcp::resolver::query query(host, std::to_string(port));
tcp::resolver::iterator iter = resolver.resolve(query);
tcp::endpoint ep = *iter;
m_sock.connect(ep);
// 读取欢迎消息
read_reply();
if (m_reply_code != 220) {
std::cerr << "FTP server is not available." << std::endl;
return;
}
// 登录
send_command("USER " + user);
read_reply();
if (m_reply_code == 331) {
send_command("PASS " + password);
read_reply();
}
if (m_reply_code != 230) {
std::cerr << "Invalid username or password." << std::endl;
return;
}
// 进入被动模式
send_command("PASV");
read_reply();
if (m_reply_code != 227) {
std::cerr << "Failed to enter passive mode." << std::endl;
return;
}
uint16_t data_port;
if (!parse_pasv_reply(m_reply_msg, data_port)) {
std::cerr << "Failed to parse PASV reply." << std::endl;
return;
}
// 下载文件
std::string filename = path.substr(path.find_last_of('/') + 1);
if (!remote_path.empty()) {
filename = remote_path + "/" + filename;
}
send_command("TYPE I");
read_reply();
if (m_reply_code != 200) {
std::cerr << "Failed to set binary mode." << std::endl;
return;
}
send_command("RETR " + filename);
read_reply();
if (m_reply_code != 150) {
std::cerr << "Failed to start download." << std::endl;
return;
}
tcp::socket data_sock(m_sock.get_io_service());
tcp::endpoint data_ep = tcp::endpoint(m_sock.remote_endpoint().address(), data_port);
data_sock.connect(data_ep);
boost::system::error_code ec;
boost::asio::streambuf buf;
boost::asio::read(data_sock, buf, ec);
data_sock.close();
std::ofstream outfile(local_path, std::ios::out | std::ios::binary);
outfile.write(boost::asio::buffer_cast<const char*>(buf.data()), buf.size());
outfile.close();
read_reply();
if (m_reply_code != 226) {
std::cerr << "Failed to complete download." << std::endl;
return;
}
}
private:
bool parse_url(const std::string& url, std::string& host, uint16_t& port, std::string& path) {
size_t pos = url.find("://");
if (pos == std::string::npos) {
return false;
}
std::string protocol = url.substr(0, pos);
if (protocol != "ftp") {
return false;
}
url = url.substr(pos + 3);
pos = url.find('/');
if (pos == std::string::npos) {
return false;
}
host = url.substr(0, pos);
url = url.substr(pos);
pos = host.find(':');
if (pos != std::string::npos) {
port = std::stoi(host.substr(pos + 1));
host = host.substr(0, pos);
}
path = url;
return true;
}
bool parse_pasv_reply(const std::string& reply, uint16_t& port) {
size_t pos1 = reply.find('(');
size_t pos2 = reply.find(')');
if (pos1 == std::string::npos || pos2 == std::string::npos) {
return false;
}
std::string data_str = reply.substr(pos1 + 1, pos2 - pos1 - 1);
std::vector<std::string> parts;
boost::split(parts, data_str, boost::is_any_of(","));
if (parts.size() != 6) {
return false;
}
uint16_t high_byte = std::stoi(parts[4]);
uint16_t low_byte = std::stoi(parts[5]);
port = high_byte * 256 + low_byte;
return true;
}
void send_command(const std::string& cmd) {
std::cout << "--> " << cmd << std::endl;
boost::asio::write(m_sock, boost::asio::buffer(cmd + "\r\n"));
}
void read_reply() {
m_reply_msg.clear();
boost::asio::streambuf buf;
boost::asio::read_until(m_sock, buf, "\r\n");
std::string line = boost::asio::buffer_cast<const char*>(buf.data());
m_reply_msg += line;
std::cout << "<-- " << line;
if (line.size() < 3 || line[3] != '-') {
m_reply_code = std::stoi(line.substr(0, 3));
}
else {
std::string cont;
do {
boost::asio::read_until(m_sock, buf, "\r\n");
line = boost::asio::buffer_cast<const char*>(buf.data());
m_reply_msg += line;
std::cout << "<-- " << line;
cont = line.substr(4);
} while (line.size() >= 4 && line[3] == '-' && !cont.empty());
m_reply_code = std::stoi(m_reply_msg.substr(0, 3));
}
}
tcp::socket m_sock;
int m_reply_code;
std::string m_reply_msg;
};
int main(int argc, char* argv[]) {
if (argc < 6) {
std::cerr << "Usage: " << argv[0] << " URL USERNAME PASSWORD REMOTE_PATH LOCAL_PATH" << std::endl;
return 1;
}
io_service ios;
ftp_client client(ios);
client.download(argv[1], argv[2], argv[3], argv[4], argv[5]);
return 0;
}
```
使用方式如下:
```
./ftp_client ftp://ftp.example.com/file.zip username password /path/to/remote/file.zip /path/to/local/file.zip
```
其中,`ftp://ftp.example.com/file.zip` 是要下载的文件的URL,`username` 和 `password` 是FTP服务器的登录凭据,`/path/to/remote/file.zip` 是文件在FTP服务器上的路径,`/path/to/local/file.zip` 是文件在本地保存的路径。
阅读全文
相关推荐
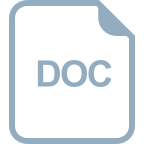
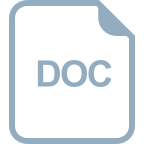
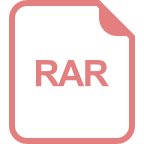
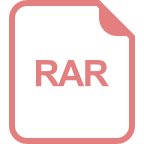
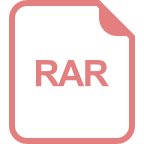
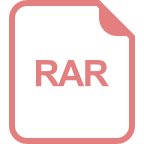
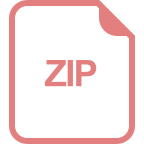
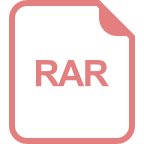
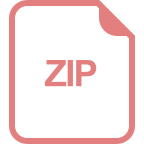
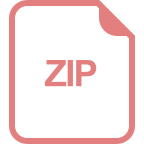
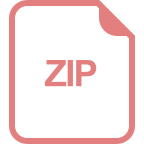
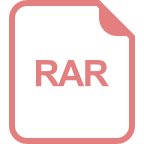
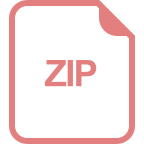
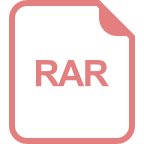
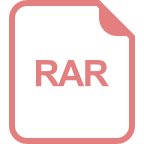
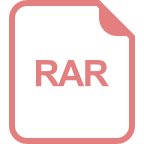

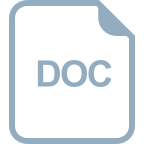