springboot vue前后端分离中,邮箱验证码
时间: 2023-12-29 10:17:49 浏览: 32
在 Spring Boot Vue 前后端分离的项目中,实现邮箱验证码可以分为以下几个步骤:
1. 前端页面中添加输入邮箱地址的表单,以及获取验证码的按钮。
2. 前端发送请求到后端,调用后端的接口生成验证码并且发送到用户的邮箱中。
3. 后端接收到前端发送的请求后,生成验证码,并且调用 JavaMailSender 发送邮件。
4. 用户在自己的邮箱中查看验证码,并在前端页面中输入该验证码。
5. 前端将用户输入的验证码和邮箱地址发送到后端进行验证。
6. 后端接收到前端发送的验证码和邮箱地址后,进行验证,验证通过则返回成功消息,否则返回错误信息。
以下是一个简单的示例代码:
前端代码:
```html
<!-- 表单 -->
<form>
<div>
<label for="email">Email:</label>
<input type="text" id="email" />
</div>
<div>
<button type="button" @click="sendVerificationCode">Send Verification Code</button>
</div>
<div>
<label for="verificationCode">Verification Code:</label>
<input type="text" id="verificationCode" />
</div>
<div>
<button type="button" @click="verifyCode">Verify Code</button>
</div>
</form>
```
```javascript
// 发送验证码
function sendVerificationCode() {
const email = document.getElementById('email').value;
axios.post('/api/sendVerificationCode', { email })
.then(response => {
console.log(response.data);
alert('Verification code sent!');
})
.catch(error => {
console.error(error);
alert('Failed to send verification code!');
});
}
// 验证验证码
function verifyCode() {
const email = document.getElementById('email').value;
const verificationCode = document.getElementById('verificationCode').value;
axios.post('/api/verifyCode', { email, verificationCode })
.then(response => {
console.log(response.data);
alert('Verification succeeded!');
})
.catch(error => {
console.error(error);
alert('Verification failed!');
});
}
```
后端代码:
```java
@RestController
@RequestMapping("/api")
public class VerificationCodeController {
@Autowired
private JavaMailSender mailSender;
private final Map<String, String> verificationMap = new ConcurrentHashMap<>();
@PostMapping("/sendVerificationCode")
public ResponseEntity<String> sendVerificationCode(@RequestBody Map<String, String> request) {
String email = request.get("email");
String verificationCode = generateVerificationCode();
sendEmail(email, verificationCode);
verificationMap.put(email, verificationCode);
return ResponseEntity.ok().body("Verification code sent!");
}
@PostMapping("/verifyCode")
public ResponseEntity<String> verifyCode(@RequestBody Map<String, String> request) {
String email = request.get("email");
String verificationCode = request.get("verificationCode");
if (verificationCode.equals(verificationMap.get(email))) {
verificationMap.remove(email);
return ResponseEntity.ok().body("Verification succeeded!");
} else {
return ResponseEntity.badRequest().body("Verification failed!");
}
}
private String generateVerificationCode() {
// 生成验证码的逻辑
}
private void sendEmail(String email, String verificationCode) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(email);
message.setSubject("Verification Code");
message.setText("Your verification code is: " + verificationCode);
mailSender.send(message);
}
}
```
需要注意的是,该示例代码中并没有对邮箱地址进行验证和格式化,实际项目中需要对邮箱地址进行验证和格式化,以保证发送邮件的准确性。
相关推荐
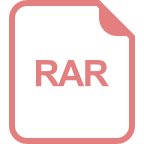
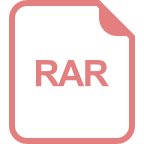














