winform调用摄像头扫描识别条形码,随后将条码id显示在文本框里面
时间: 2023-10-25 08:08:55 浏览: 189
好的,这个问题涉及到以下几个步骤:
1. 引入摄像头设备并进行初始化。
2. 开启摄像头进行扫描条形码。
3. 对扫描到的条形码进行解码。
4. 将解码后的条形码ID显示在WinForm窗体中的文本框中。
下面是一个简单的实现过程:
1. 引入摄像头设备并进行初始化。
```c#
using System;
using System.Windows.Forms;
using ZXing;
using ZXing.Common;
using AForge.Video;
using AForge.Video.DirectShow;
namespace BarcodeScanner
{
public partial class Form1 : Form
{
private FilterInfoCollection videoDevices;
private VideoCaptureDevice videoSource;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
if (videoDevices.Count > 0)
{
foreach (FilterInfo device in videoDevices)
{
comboBox1.Items.Add(device.Name);
}
comboBox1.SelectedIndex = 0;
}
else
{
MessageBox.Show("未检测到摄像头设备!");
}
}
private void button1_Click(object sender, EventArgs e)
{
videoSource = new VideoCaptureDevice(videoDevices[comboBox1.SelectedIndex].MonikerString);
videoSource.NewFrame += new NewFrameEventHandler(videoSource_NewFrame);
videoSource.Start();
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
if (videoSource != null && videoSource.IsRunning)
{
videoSource.SignalToStop();
videoSource = null;
}
}
}
}
```
2. 开启摄像头进行扫描条形码。
```c#
private void videoSource_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
Bitmap bitmap = (Bitmap)eventArgs.Frame.Clone();
pictureBox1.Image = bitmap;
BarcodeReader reader = new BarcodeReader();
Result result = reader.Decode(bitmap);
if (result != null)
{
string barcode = result.Text;
textBox1.Invoke(new Action(() => textBox1.Text = barcode));
}
}
```
3. 对扫描到的条形码进行解码。
```c#
BarcodeReader reader = new BarcodeReader();
Result result = reader.Decode(bitmap);
if (result != null)
{
string barcode = result.Text;
textBox1.Invoke(new Action(() => textBox1.Text = barcode));
}
```
4. 将解码后的条形码ID显示在WinForm窗体中的文本框中。
```c#
textBox1.Invoke(new Action(() => textBox1.Text = barcode));
```
最后,需要注意的是,此实现仅为简单示例,实际应用中还需要考虑到异常处理、线程安全等问题。
阅读全文
相关推荐



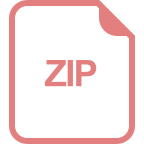
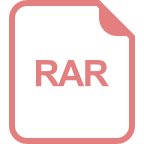
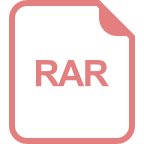
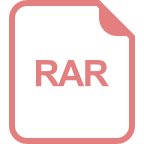
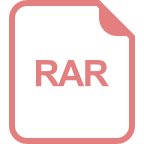
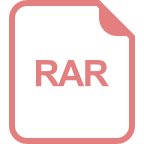
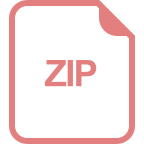
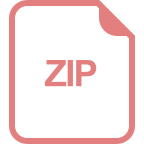



