winform调用摄像头扫描识别条形码,将条码id显示在文本框里面
时间: 2023-10-25 08:08:55 浏览: 213
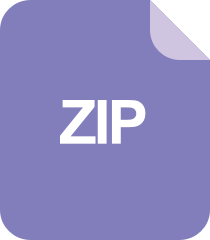
从WinForm / WPF应用程序中的网络摄像头扫描条形码
好的,这个问题可以分为以下几个步骤来实现:
1. 引入相关的命名空间
在 Winform 窗体中使用摄像头扫描条形码,需要使用到 `System.Drawing` 和 `System.Windows.Forms` 命名空间,因此需要在代码文件中引入这两个命名空间:
```csharp
using System.Drawing;
using System.Windows.Forms;
```
2. 创建摄像头控件
在 Winform 窗体中,可以使用 `PictureBox` 控件来显示摄像头捕捉到的图像,因此需要创建一个 `PictureBox` 控件,用于显示摄像头的图像:
```csharp
private PictureBox pictureBox1;
```
3. 初始化摄像头控件
在窗体的 `Load` 事件中初始化摄像头控件,并启动摄像头:
```csharp
private void Form1_Load(object sender, EventArgs e)
{
// 初始化摄像头控件
pictureBox1 = new PictureBox();
pictureBox1.SizeMode = PictureBoxSizeMode.StretchImage;
pictureBox1.Dock = DockStyle.Fill;
Controls.Add(pictureBox1);
// 启动摄像头
VideoCaptureDevice videoSource = new VideoCaptureDevice();
FilterInfoCollection videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
if (videoDevices.Count > 0)
{
videoSource = new VideoCaptureDevice(videoDevices[0].MonikerString);
videoSource.NewFrame += new NewFrameEventHandler(videoSource_NewFrame);
videoSource.Start();
}
}
```
其中,`videoSource_NewFrame` 是摄像头捕捉到新图像时的回调函数,可以在该函数中对图像进行处理。
4. 处理摄像头图像
在 `videoSource_NewFrame` 回调函数中,可以对摄像头捕捉到的图像进行处理。首先,需要使用 `ZXing.Net` 库来识别条形码:
```csharp
private void videoSource_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
Bitmap bitmap = (Bitmap)eventArgs.Frame.Clone();
BarcodeReader reader = new BarcodeReader();
Result result = reader.Decode(bitmap);
if (result != null)
{
string barcode = result.Text;
// 将条码id显示在文本框中
textBox1.Text = barcode;
}
pictureBox1.Image = bitmap;
}
```
其中,`BarcodeReader` 是 `ZXing.Net` 库中用于识别条形码的类,`reader.Decode(bitmap)` 方法可以识别传入的图像中的条形码,并返回一个 `Result` 对象。如果识别成功,可以从 `Result.Text` 属性中获取条形码的内容。
最后,在窗体中添加一个 `TextBox` 控件,用于显示条形码的内容:
```csharp
private TextBox textBox1;
```
完整的代码如下:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using AForge.Video.DirectShow;
using ZXing;
namespace BarcodeScanner
{
public partial class Form1 : Form
{
private PictureBox pictureBox1;
private TextBox textBox1;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
// 初始化摄像头控件
pictureBox1 = new PictureBox();
pictureBox1.SizeMode = PictureBoxSizeMode.StretchImage;
pictureBox1.Dock = DockStyle.Fill;
Controls.Add(pictureBox1);
// 初始化文本框控件
textBox1 = new TextBox();
textBox1.Dock = DockStyle.Bottom;
textBox1.ReadOnly = true;
Controls.Add(textBox1);
// 启动摄像头
VideoCaptureDevice videoSource = new VideoCaptureDevice();
FilterInfoCollection videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
if (videoDevices.Count > 0)
{
videoSource = new VideoCaptureDevice(videoDevices[0].MonikerString);
videoSource.NewFrame += new NewFrameEventHandler(videoSource_NewFrame);
videoSource.Start();
}
}
private void videoSource_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
Bitmap bitmap = (Bitmap)eventArgs.Frame.Clone();
BarcodeReader reader = new BarcodeReader();
Result result = reader.Decode(bitmap);
if (result != null)
{
string barcode = result.Text;
// 将条码id显示在文本框中
textBox1.Text = barcode;
}
pictureBox1.Image = bitmap;
}
}
}
```
注意:在使用 `ZXing.Net` 库时,需要先从 NuGet 中安装 `ZXing.Net` 包。
阅读全文
相关推荐
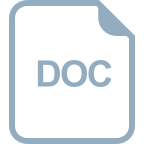
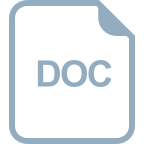


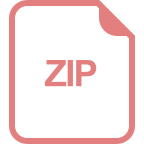
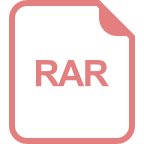
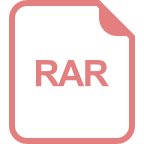
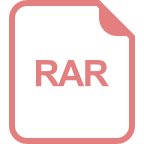
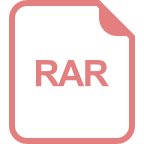
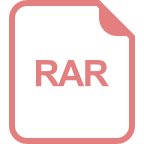
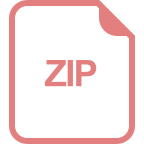
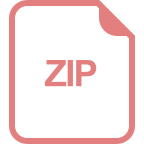





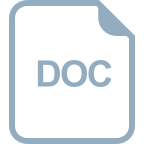