winform打开本地摄像头识别条形码在文本框中,完整代码及组件
时间: 2023-10-17 11:05:12 浏览: 113
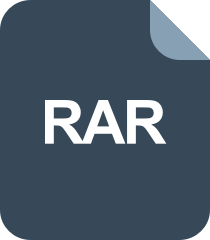
摄像头读取条形码

以下是一个基于WinForm的条形码识别程序的完整代码及组件。
首先需要引入以下命名空间:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using AForge.Video;
using AForge.Video.DirectShow;
using ZXing;
```
然后在Form的类中定义以下字段:
```csharp
private FilterInfoCollection videoDevices;
private VideoCaptureDevice videoDevice;
private Bitmap currentFrame;
private BarcodeReader barcodeReader;
```
在Form的构造函数中初始化这些字段:
```csharp
public Form1()
{
InitializeComponent();
// 初始化摄像头设备
videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
if (videoDevices.Count != 0)
{
videoDevice = new VideoCaptureDevice(videoDevices[0].MonikerString);
videoDevice.NewFrame += new NewFrameEventHandler(videoDevice_NewFrame);
videoDevice.Start();
}
// 初始化条形码读取器
barcodeReader = new BarcodeReader();
}
```
在Form的FormClosing事件中停止摄像头设备:
```csharp
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
if (videoDevice != null && videoDevice.IsRunning)
{
videoDevice.SignalToStop();
videoDevice.WaitForStop();
videoDevice = null;
}
}
```
在Form中添加一个PictureBox控件,并在videoDevice_NewFrame事件中实时显示摄像头捕获的画面:
```csharp
private void videoDevice_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
// 显示摄像头捕获的画面
currentFrame = (Bitmap)eventArgs.Frame.Clone();
pictureBox1.Image = currentFrame;
}
```
最后,在Form中添加一个TextBox控件,并在Timer的Tick事件中实现条形码识别并将结果显示在TextBox中:
```csharp
private void timer1_Tick(object sender, EventArgs e)
{
// 识别条形码并显示结果
if (currentFrame != null)
{
Result result = barcodeReader.Decode(currentFrame);
if (result != null)
{
textBox1.Text = result.Text;
}
}
}
```
完整代码如下:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using AForge.Video;
using AForge.Video.DirectShow;
using ZXing;
namespace BarcodeReader
{
public partial class Form1 : Form
{
private FilterInfoCollection videoDevices;
private VideoCaptureDevice videoDevice;
private Bitmap currentFrame;
private BarcodeReader barcodeReader;
public Form1()
{
InitializeComponent();
// 初始化摄像头设备
videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
if (videoDevices.Count != 0)
{
videoDevice = new VideoCaptureDevice(videoDevices[0].MonikerString);
videoDevice.NewFrame += new NewFrameEventHandler(videoDevice_NewFrame);
videoDevice.Start();
}
// 初始化条形码读取器
barcodeReader = new BarcodeReader();
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
if (videoDevice != null && videoDevice.IsRunning)
{
videoDevice.SignalToStop();
videoDevice.WaitForStop();
videoDevice = null;
}
}
private void videoDevice_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
// 显示摄像头捕获的画面
currentFrame = (Bitmap)eventArgs.Frame.Clone();
pictureBox1.Image = currentFrame;
}
private void timer1_Tick(object sender, EventArgs e)
{
// 识别条形码并显示结果
if (currentFrame != null)
{
Result result = barcodeReader.Decode(currentFrame);
if (result != null)
{
textBox1.Text = result.Text;
}
}
}
}
}
```
需要注意的是,以上代码中使用了AForge和ZXing两个第三方库,需要通过NuGet安装。可以在Visual Studio中打开“工具”->“NuGet包管理器”->“管理解决方案的NuGet包”窗口,搜索并安装AForge和ZXing。
阅读全文
相关推荐







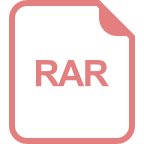
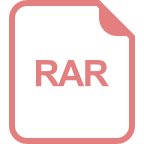
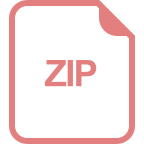
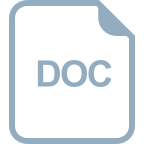


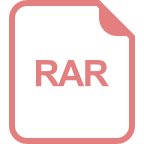
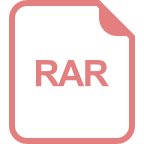
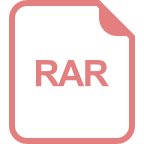