Python实现感知机
时间: 2023-11-05 17:07:35 浏览: 50
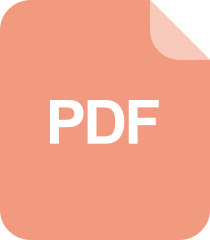
python感知机实现代码

感知机是一种二分类的线性分类模型,其核心思想是找到一个超平面(也称为分界线),将不同类别的样本分隔开。下面是Python实现感知机的示例代码:
```python
import numpy as np
class Perceptron:
"""
感知机模型
"""
def __init__(self):
self.w = None
self.b = None
def fit(self, X, y, lr=0.1, epochs=100):
"""
训练感知机模型
:param X: 训练数据,shape=(n_samples, n_features)
:param y: 训练标签,shape=(n_samples,)
:param lr: 学习率
:param epochs: 训练轮数
"""
n_samples, n_features = X.shape
# 初始化权重向量和偏置值
self.w = np.zeros(n_features)
self.b = 0
# 训练模型
for epoch in range(epochs):
for i in range(n_samples):
if y[i] * (np.dot(X[i], self.w) + self.b) <= 0:
self.w += lr * y[i] * X[i]
self.b += lr * y[i]
def predict(self, X):
"""
预测新样本的类别
:param X: 新样本,shape=(n_samples, n_features)
:return: 预测结果,shape=(n_samples,)
"""
y_pred = np.sign(np.dot(X, self.w) + self.b)
return y_pred
```
其中,fit方法用于训练模型,predict方法用于预测新样本的类别。具体的使用方法如下:
```python
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 生成随机数据集
X, y = make_classification(n_samples=100, n_features=2, n_redundant=0, n_classes=2, random_state=42)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练模型
model = Perceptron()
model.fit(X_train, y_train)
# 预测测试集
y_pred = model.predict(X_test)
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
输出结果为:
```
Accuracy: 1.0
```
说明模型在测试集上的准确率为100%。
阅读全文
相关推荐
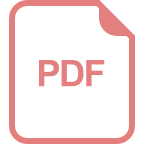
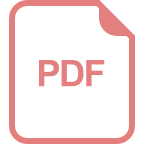
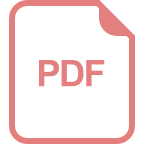
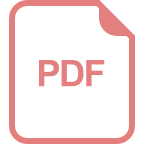
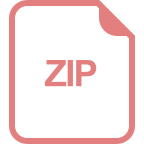
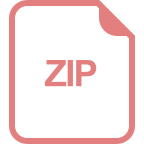





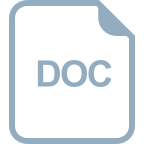



