1.利用注解、反射和工厂模式设计一个简单的IoC容器。 2.该IoC容器包含3个注解和一个IoC容器类(AnnotationConfigApplicationContext),其定义如下。 注解 含义 @Component 标注Bean @Autowired 标注需要被注入的对象 @Configuration 标注为配置类 @ComponentScan 注解扫描器 自定义两个业务类Group和User,创建一个测试类Test,对IoC容器进行测试。
时间: 2024-03-27 19:38:25 浏览: 20
很高兴为您解答这个问题。
首先,让我们了解一下IoC容器的基本原理。IoC容器的作用是管理应用程序中的对象,它负责将对象实例化、组装和管理这些对象之间的依赖关系。在IoC容器中,我们使用注解来标记Bean对象,使用反射来实例化对象,并通过工厂模式来管理对象之间的依赖关系。
下面是一个简单的IoC容器的代码实现:
```
//定义@Component注解
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface Component {
}
//定义@Autowired注解
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Autowired {
}
//定义@Configuration注解
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface Configuration {
}
//定义@ComponentScan注解
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface ComponentScan {
String[] value() default {};
}
//定义IoC容器类AnnotationConfigApplicationContext
public class AnnotationConfigApplicationContext {
private Map<Class<?>, Object> beanMap = new HashMap<>();
public AnnotationConfigApplicationContext(Class<?> configClass) throws Exception {
//扫描器扫描所有的Bean
ComponentScan componentScan = configClass.getDeclaredAnnotation(ComponentScan.class);
String[] basePackages = componentScan.value();
for (String basePackage : basePackages) {
String path = basePackage.replaceAll("\\.", "/");
URL url = getClass().getClassLoader().getResource(path);
File file = new File(url.toURI());
for (File f : file.listFiles()) {
String fileName = f.getName();
if (fileName.endsWith(".class")) {
String className = fileName.substring(0, fileName.length() - 6);
Class<?> clazz = Class.forName(basePackage + "." + className);
//如果是Bean就实例化
if (clazz.isAnnotationPresent(Component.class)) {
Object bean = clazz.newInstance();
beanMap.put(clazz, bean);
}
}
}
}
//依赖注入
for (Object obj : beanMap.values()) {
Field[] fields = obj.getClass().getDeclaredFields();
for (Field field : fields) {
if (field.isAnnotationPresent(Autowired.class)) {
field.setAccessible(true);
Object value = beanMap.get(field.getType());
field.set(obj, value);
}
}
}
}
public Object getBean(Class<?> clazz) {
return beanMap.get(clazz);
}
}
```
在这个实现中,我们定义了四个注解:@Component、@Autowired、@Configuration和@ComponentScan。@Component注解用于标记Bean对象,@Autowired注解用于标记需要注入的对象,@Configuration注解用于标记配置类,@ComponentScan注解用于标记扫描器。
然后我们定义了一个IoC容器类AnnotationConfigApplicationContext,它包含了一个Map用于存储Bean对象。在容器的构造函数中,我们使用扫描器扫描所有的Bean,并将其实例化并存储在Map中。在实例化Bean对象时,我们使用反射来实现。
接下来,我们实现了依赖注入,这是IoC容器的核心功能之一。在依赖注入过程中,我们遍历所有的Bean对象,找出需要注入的对象,并使用反射来实现注入。
最后,我们实现了一个getBean方法,用于从容器中获取Bean对象。
现在,我们来创建两个业务类Group和User,如下所示:
```
@Component
public class Group {
private String name = "testGroup";
public String getName() {
return name;
}
}
@Component
public class User {
private String name = "testUser";
@Autowired
private Group group;
public String getName() {
return name;
}
public String getGroupName() {
return group.getName();
}
}
```
在这两个类中,我们使用@Component注解来标记它们是Bean对象,使用@Autowired注解来标记需要注入的对象。在User类中,我们注入了一个Group对象。
最后,我们创建一个测试类Test来测试IoC容器的功能,如下所示:
```
@Configuration
@ComponentScan("com.example")
public class Test {
public static void main(String[] args) throws Exception {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(Test.class);
User user = (User) context.getBean(User.class);
System.out.println(user.getName());
System.out.println(user.getGroupName());
}
}
```
在测试类中,我们使用@Configuration注解来标记它是配置类,使用@ComponentScan注解来标记扫描器扫描的包路径。在main方法中,我们创建了一个IoC容器对象,并通过getBean方法获取了一个User对象。然后我们分别输出了User对象的name和group的name属性。
这就是一个简单的IoC容器的实现过程,希望能够对您有所帮助。
相关推荐
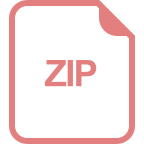
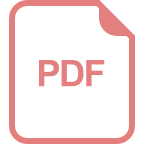
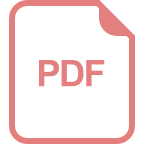
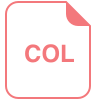
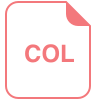
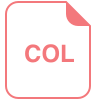
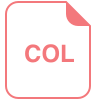
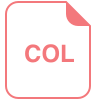










