写一段电商购物车代码
时间: 2023-07-10 11:06:19 浏览: 167
下面是一个简单的电商购物车代码示例:
```python
# 定义购物车类
class ShoppingCart:
def __init__(self):
self.items = {}
# 添加商品到购物车
def add_item(self, product, quantity):
if product in self.items:
self.items[product] += quantity
else:
self.items[product] = quantity
# 从购物车中删除商品
def remove_item(self, product):
if product in self.items:
del self.items[product]
# 更新购物车中商品数量
def update_quantity(self, product, quantity):
if product in self.items:
self.items[product] = quantity
# 获取购物车中商品总数
def get_total_quantity(self):
return sum(self.items.values())
# 获取购物车中商品总价
def get_total_price(self, price_table):
total_price = 0
for product, quantity in self.items.items():
total_price += price_table[product] * quantity
return total_price
# 定义商品和价格表
products = ["iphone", "ipad", "macbook"]
prices = {"iphone": 6999, "ipad": 3999, "macbook": 9999}
# 创建购物车实例并添加商品
cart = ShoppingCart()
cart.add_item("iphone", 2)
cart.add_item("ipad", 1)
# 打印购物车信息
print("购物车中商品数量:", cart.get_total_quantity())
print("购物车中商品总价:", cart.get_total_price(prices))
# 更新购物车中商品数量并删除商品
cart.update_quantity("iphone", 3)
cart.remove_item("ipad")
# 再次打印购物车信息
print("购物车中商品数量:", cart.get_total_quantity())
print("购物车中商品总价:", cart.get_total_price(prices))
```
这段代码定义了一个`ShoppingCart`类,实现了添加商品、删除商品、更新商品数量、获取商品总数量和总价等方法。在主程序中,我们创建了一个购物车实例并添加了两个商品,然后打印购物车中商品数量和总价。接着,我们更新了购物车中的商品数量并删除了一个商品,再次打印购物车信息。
阅读全文
相关推荐
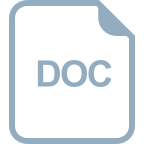


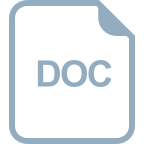
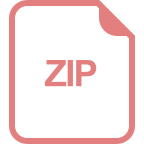
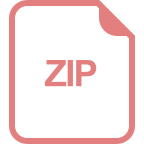
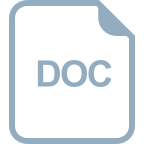
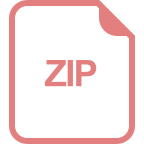
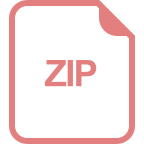
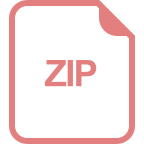
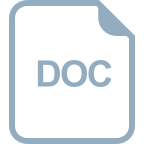
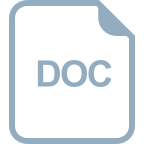
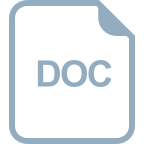
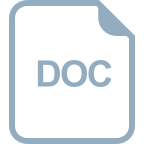

