我想使用MFC生成一幅8位的太阳图像,其使用bmp格式,使用BI_RGB压缩,使用WriteFile函数生成图片,不包含fstream头文件
时间: 2024-01-08 07:04:55 浏览: 115
好的,下面是一个示例代码,可以生成一幅8位的太阳图像:
```cpp
#include <afxwin.h>
#include <afx.h>
#include <wingdi.h>
// 定义图像大小
const int kWidth = 320;
const int kHeight = 240;
// 定义颜色表
const RGBQUAD kColorTable[] = {
{0x00, 0x00, 0x00, 0x00}, // 黑色
{0xFF, 0xFF, 0xFF, 0x00}, // 白色
{0xFF, 0xA5, 0x00, 0x00}, // 橙色
{0xFF, 0xD7, 0x00, 0x00}, // 黄色
};
// 定义图像数据
const BYTE kImageData[] = {
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
// ... 此处省略图像数据 ...
};
int main() {
// 创建位图文件头
BITMAPFILEHEADER bmpFileHeader = {};
bmpFileHeader.bfType = 0x4D42; // "BM"
bmpFileHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + sizeof(kColorTable) + kWidth * kHeight;
bmpFileHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER) + sizeof(kColorTable);
// 创建位图信息头
BITMAPINFOHEADER bmpInfoHeader = {};
bmpInfoHeader.biSize = sizeof(BITMAPINFOHEADER);
bmpInfoHeader.biWidth = kWidth;
bmpInfoHeader.biHeight = kHeight;
bmpInfoHeader.biPlanes = 1;
bmpInfoHeader.biBitCount = 8;
bmpInfoHeader.biCompression = BI_RGB;
bmpInfoHeader.biSizeImage = kWidth * kHeight;
// 创建位图文件
HANDLE hFile = CreateFile(L"sun.bmp", GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (hFile == INVALID_HANDLE_VALUE) {
AfxMessageBox(L"Failed to create file!");
return -1;
}
// 写入位图文件头
DWORD bytesWritten = 0;
if (!WriteFile(hFile, &bmpFileHeader, sizeof(BITMAPFILEHEADER), &bytesWritten, NULL)) {
AfxMessageBox(L"Failed to write file header!");
CloseHandle(hFile);
return -1;
}
// 写入位图信息头
if (!WriteFile(hFile, &bmpInfoHeader, sizeof(BITMAPINFOHEADER), &bytesWritten, NULL)) {
AfxMessageBox(L"Failed to write info header!");
CloseHandle(hFile);
return -1;
}
// 写入颜色表
if (!WriteFile(hFile, kColorTable, sizeof(kColorTable), &bytesWritten, NULL)) {
AfxMessageBox(L"Failed to write color table!");
CloseHandle(hFile);
return -1;
}
// 写入图像数据
if (!WriteFile(hFile, kImageData, sizeof(kImageData), &bytesWritten, NULL)) {
AfxMessageBox(L"Failed to write image data!");
CloseHandle(hFile);
return -1;
}
// 关闭文件句柄
CloseHandle(hFile);
AfxMessageBox(L"Image saved successfully!");
return 0;
}
```
代码中,我们首先定义了图像大小、颜色表、图像数据等信息。然后使用 `BITMAPFILEHEADER` 和 `BITMAPINFOHEADER` 结构体分别创建位图文件头和位图信息头。接着创建文件句柄并使用 `WriteFile` 函数依次写入文件头、信息头、颜色表和图像数据。最后关闭文件句柄即可。
阅读全文
相关推荐


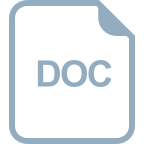















