def collate_fn(batch: List[Tuple[torch.Tensor, torch.Tensor]]) -> Tuple[torch.Tensor, torch.Tensor]: """数据处理函数""" texts = [item[0] for item in batch] labels = [item[1] for item in batch] max_len = max([len(text) for text in texts]) texts = [torch.cat([text, torch.tensor([0] * (max_len - len(text)))]) for text in texts] labels = [torch.cat([label, torch.tensor([-1] * (max_len - len(label)))]) for label in labels] mask = torch.tensor([[1] * len(text) + [0] * (max_len - len(text)) for text in texts]) return torch.stack(texts), torch.stack(labels), mask
时间: 2023-07-01 12:04:02 浏览: 75
这是一个用于PyTorch中的数据处理函数,输入参数为一个由Tuple组成的List。每个Tuple包含两个Tensor,第一个Tensor为文本数据,第二个Tensor为标签数据。该函数通过以下步骤处理数据:
1. 将所有文本数据存入一个列表texts中,将所有标签数据存入一个列表labels中。
2. 找到texts中最长的文本,并将所有文本的长度都调整为最长文本的长度。
3. 将texts列表中的每一个Tensor都用0进行填充,使其长度与最长文本相同。
4. 将labels列表中的每一个Tensor都用-1进行填充,使其长度与最长文本相同。
5. 创建一个掩码矩阵mask,用1表示文本数据的有效部分,用0表示文本数据的填充部分。
6. 返回一个Tuple,包含三个Tensor,分别为处理后的文本数据、处理后的标签数据和掩码矩阵。
相关问题
AttributeError: module 'torch.utils' has no attribute 'collate_fn'
AttributeError: module 'torch.utils' has no attribute 'collate_fn'是一个错误提示,意味着在torch.utils模块中没有名为collate_fn的属性。这通常发生在使用torch.utils.data.DataLoader时,因为collate_fn是DataLoader的一个参数。
DataLoader是PyTorch中用于加载数据的实用程序类。它可以将数据集封装成一个可迭代的对象,方便进行批量处理和并行加载数据。在使用DataLoader时,可以通过collate_fn参数指定一个函数来自定义数据的批量处理方式。
如果你遇到了这个错误,可能有以下几种原因:
1. 你可能拼写错误,应该检查拼写是否正确。
2. 你可能使用了过时的版本的PyTorch,建议升级到最新版本。
3. 你可能没有正确导入所需的模块或函数。
为了解决这个问题,你可以尝试以下几个步骤:
1. 确保你的PyTorch版本是最新的,可以通过运行`pip install torch -U`来升级。
2. 检查你的代码中是否正确导入了torch.utils.data.DataLoader和其他相关模块。
3. 检查你的代码中是否正确使用了collate_fn参数,并确保拼写正确。
4. 如果以上步骤都没有解决问题,可以尝试重新安装PyTorch。
torch.utils.data.DataLoader中collate_fn
在PyTorch中,torch.utils.data.DataLoader中的collate_fn参数用于指定如何将一个batch的数据样本整合成一个batch的张量。默认情况下,collate_fn使用torch.stack函数将数据样本堆叠在一起。如果数据样本具有不同的大小,则需要自定义collate_fn函数来处理。
例如,如果数据样本是一个元组,其中第一个元素是图像张量,第二个元素是标签张量,则可以使用以下自定义collate_fn函数:
```python
def custom_collate_fn(batch):
images = []
labels = []
for image, label in batch:
images.append(image)
labels.append(label)
images = torch.stack(images, dim=0)
labels = torch.tensor(labels)
return images, labels
```
相关推荐
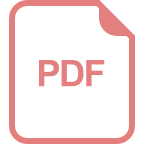
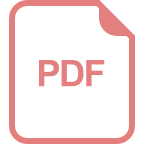













