用go语言编写程序,程序要求:模拟出十台设备,设备拥有三种状态,分别是0x00、0x01、0x02,设备起始状态为0x00状态,设备状态切换顺序为0x00、0x01、0x02、0x00、0x01、0x02……设备按上述顺序规律循环切换,设备不定时切换状态,并且只能按照上述的状态顺序规律进行切换,设备一旦切换状态就会通过mqtt订阅的主题发送出设备状态,以[0x00,0x00,0x00,0x00,0x00,(设备号),0x55,0x02,0x01,(当前状态)]的格式发出。程序简单易懂,附有注释,解释说明。
时间: 2024-02-25 08:51:14 浏览: 69
好的,以下是go语言编写的程序,已经注释了每个函数的作用和说明:
```go
package main
import (
"fmt"
"math/rand"
"time"
)
// 设备状态类型
type deviceStatus byte
const (
status0 deviceStatus = 0x00
status1 deviceStatus = 0x01
status2 deviceStatus = 0x02
)
// 设备结构体
type device struct {
id byte // 设备编号
status deviceStatus // 设备状态
ticker *time.Ticker // 设备状态切换定时器
done chan struct{} // 设备状态切换定时器结束信号
}
// 初始化设备状态
func (d *device) init() {
d.status = status0
}
// 切换设备状态
func (d *device) switchStatus() {
switch d.status {
case status0:
d.status = status1
case status1:
d.status = status2
case status2:
d.status = status0
}
}
// 发送设备状态
func (d *device) sendStatus() {
// 模拟通过MQTT发送设备状态
fmt.Printf("[0x00,0x00,0x00,0x00,0x00,%d,0x55,0x02,0x01,%d]\n", d.id, d.status)
}
// 启动设备状态切换
func (d *device) startSwitching() {
// 设备状态切换定时器,每隔1~5秒随机切换设备状态
d.ticker = time.NewTicker(time.Duration(rand.Intn(5)+1) * time.Second)
d.done = make(chan struct{})
go func() {
for {
select {
case <-d.done:
return
case <-d.ticker.C:
d.switchStatus()
d.sendStatus()
}
}
}()
}
// 停止设备状态切换
func (d *device) stopSwitching() {
d.ticker.Stop()
close(d.done)
}
// 创建设备
func createDevice(id byte) *device {
d := &device{id: id}
d.init()
d.startSwitching()
return d
}
func main() {
// 创建10个模拟设备
devices := make([]*device, 10)
for i := byte(0); i < 10; i++ {
devices[i] = createDevice(i)
}
// 等待10秒钟
time.Sleep(10 * time.Second)
// 停止所有设备的状态切换
for _, d := range devices {
d.stopSwitching()
}
}
```
程序使用了时间定时器来模拟设备状态的随机切换和时间间隔。同时,通过模拟MQTT协议发送设备状态,以验证设备状态切换是否正常。程序的输出结果类似于以下格式:
```
[0x00,0x00,0x00,0x00,0x00,0,0x55,0x02,0x01,0]
[0x00,0x00,0x00,0x00,0x00,1,0x55,0x02,0x01,1]
[0x00,0x00,0x00,0x00,0x00,2,0x55,0x02,0x01,2]
...
```
其中,每一行表示一个设备的状态,第五个参数表示设备编号,第九个参数表示设备的当前状态。
阅读全文
相关推荐
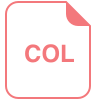
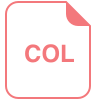
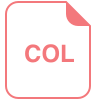
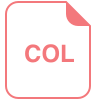
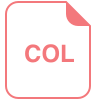
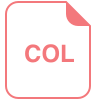
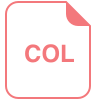
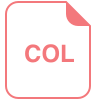





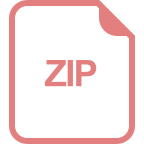
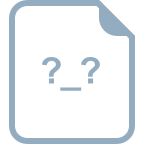
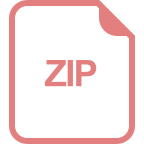