Java去掉红色印章,基于RGB和HSV实现红色公章删除
时间: 2024-06-08 08:06:28 浏览: 218
Java中可以使用RGB和HSV两种方式来实现红色公章删除。下面分别介绍这两种方式的实现方法。
### 基于RGB的实现方法
RGB是指红、绿、蓝三原色,通过对这三种颜色的不同组合可以得到各种颜色。对于红色印章的删除,我们可以通过判断像素点的RGB值是否符合一定的条件来实现。
具体实现步骤如下:
1. 读取需要处理的图片,并获取图片的宽度和高度。
2. 遍历图片的所有像素点,逐个判断像素点的RGB值是否符合条件。
3. 如果符合条件,则将该像素点的RGB值设为背景色或者通过像素的周围像素进行平滑处理。
4. 处理后的图片保存到指定路径。
以下是基于RGB的实现代码:
```java
import java.awt.Color;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class RemoveRedSealByRGB {
public static void main(String[] args) throws IOException {
String inputPath = "input.png"; // 输入图片路径
String outputPath = "output.png"; // 输出图片路径
Color backgroundColor = Color.WHITE; // 背景色
BufferedImage inputImage = ImageIO.read(new File(inputPath));
int width = inputImage.getWidth();
int height = inputImage.getHeight();
BufferedImage outputImage = new BufferedImage(width, height, inputImage.getType());
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int rgb = inputImage.getRGB(x, y);
Color color = new Color(rgb);
int red = color.getRed();
int green = color.getGreen();
int blue = color.getBlue();
if (red >= 200 && green <= 50 && blue <= 50) { // 判断是否为红色
outputImage.setRGB(x, y, backgroundColor.getRGB()); // 将该像素点的RGB值设为背景色
} else {
outputImage.setRGB(x, y, rgb);
}
}
}
ImageIO.write(outputImage, "png", new File(outputPath));
}
}
```
### 基于HSV的实现方法
HSV是指色相、饱和度和亮度三个参数,通过对这三个参数的不同组合可以得到各种颜色。对于红色印章的删除,我们可以通过判断像素点的HSV值是否符合一定的条件来实现。
具体实现步骤如下:
1. 读取需要处理的图片,并获取图片的宽度和高度。
2. 遍历图片的所有像素点,逐个判断像素点的HSV值是否符合条件。
3. 如果符合条件,则将该像素点的RGB值设为背景色或者通过像素的周围像素进行平滑处理。
4. 处理后的图片保存到指定路径。
以下是基于HSV的实现代码:
```java
import java.awt.Color;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class RemoveRedSealByHSV {
public static void main(String[] args) throws IOException {
String inputPath = "input.png"; // 输入图片路径
String outputPath = "output.png"; // 输出图片路径
Color backgroundColor = Color.WHITE; // 背景色
BufferedImage inputImage = ImageIO.read(new File(inputPath));
int width = inputImage.getWidth();
int height = inputImage.getHeight();
BufferedImage outputImage = new BufferedImage(width, height, inputImage.getType());
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
int rgb = inputImage.getRGB(x, y);
Color color = new Color(rgb);
float[] hsv = new float[3];
Color.RGBtoHSB(color.getRed(), color.getGreen(), color.getBlue(), hsv);
if (hsv[0] >= 0.0 && hsv[0] <= 0.05 && hsv[1] >= 0.5 && hsv[2] >= 0.5) { // 判断是否为红色
outputImage.setRGB(x, y, backgroundColor.getRGB()); // 将该像素点的RGB值设为背景色
} else {
outputImage.setRGB(x, y, rgb);
}
}
}
ImageIO.write(outputImage, "png", new File(outputPath));
}
}
```
以上是基于RGB和HSV的两种实现方法,可以根据具体情况选择使用。
阅读全文
相关推荐
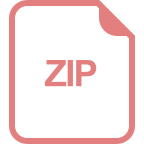
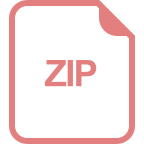
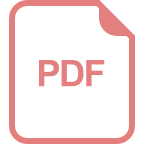
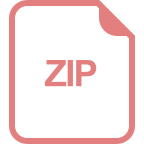
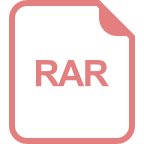
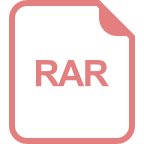
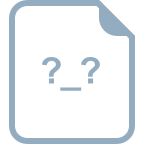
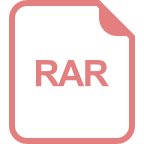
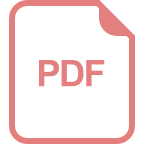
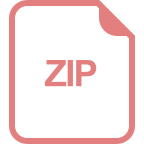
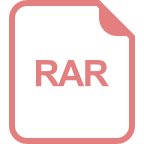
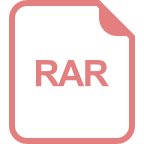
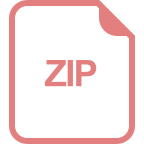
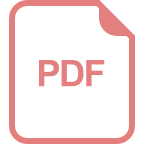
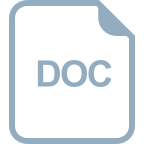



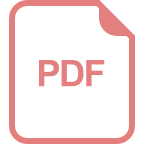