编写程序esp8266的web server 处理一个带参数的post请求
时间: 2023-11-22 19:52:32 浏览: 111
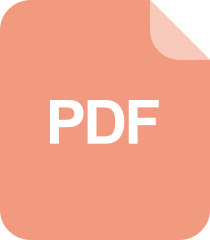
详解使用fetch发送post请求时的参数处理
好的,我可以给你提供一些思路和示例代码。在ESP8266上实现一个带参数的POST请求,可以参考以下步骤:
1. 首先需要连接WiFi网络,确保ESP8266能够访问互联网。可以使用ESP8266 Arduino Core库提供的WiFi连接函数,例如:
```
#include <ESP8266WiFi.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
void setup() {
// Connect to WiFi network
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
```
2. 接下来,需要设置HTTP请求头和请求体。可以使用ESP8266HTTPClient库发送HTTP请求,例如:
```
#include <ESP8266HTTPClient.h>
void loop() {
// Wait for WiFi connection
if (WiFi.status() != WL_CONNECTED) {
return;
}
// Create HTTP client object
HTTPClient http;
// Set request headers
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
// Set request body
String requestBody = "param1=value1¶m2=value2";
http.begin("http://your_server_url", requestBody);
// Send POST request
int httpResponseCode = http.POST(requestBody);
// Check response code
if (httpResponseCode > 0) {
String response = http.getString();
Serial.println(response);
} else {
Serial.print("Error: ");
Serial.println(httpResponseCode);
}
// Close HTTP connection
http.end();
}
```
在上面的示例代码中,设置了请求头为`Content-Type: application/x-www-form-urlencoded`,表示请求体采用`key=value`形式的参数。然后设置了请求体为`param1=value1¶m2=value2`,表示两个参数分别为`param1`和`param2`,对应的值分别为`value1`和`value2`。最后使用`http.POST(requestBody)`发送POST请求,并根据返回的状态码进行处理。
希望这些信息能够帮助你实现带参数的POST请求。
阅读全文
相关推荐
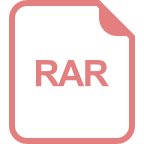
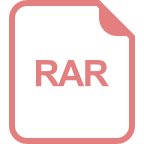
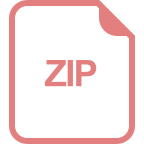
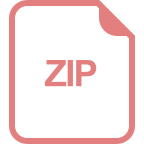
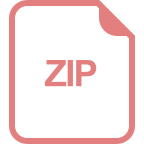
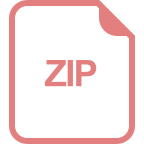
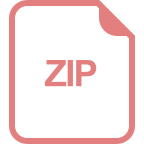
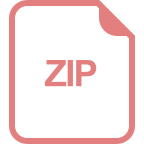
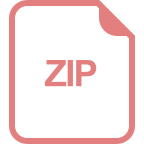
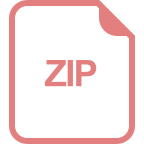
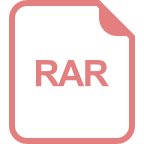
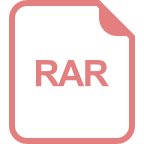
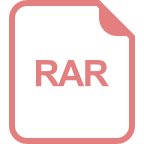
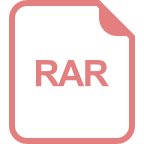
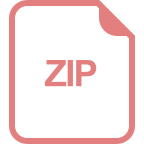
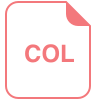