java五子棋游戏代码
时间: 2023-07-09 07:29:42 浏览: 83
以下是一个简单的 Java 五子棋游戏代码示例,包含了基本的游戏逻辑和界面实现:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Gobang extends JFrame implements ActionListener, MouseListener {
private static final int ROWS = 15; // 棋盘行数
private static final int COLS = 15; // 棋盘列数
private static final int CELL_SIZE = 30; // 棋盘格子大小
private static final int WIDTH = ROWS * CELL_SIZE + 50; // 窗口宽度
private static final int HEIGHT = COLS * CELL_SIZE + 70; // 窗口高度
private static final int MARGIN = CELL_SIZE / 2; // 棋盘边缘大小
private static final int PIECE_SIZE = CELL_SIZE - 10; // 棋子大小
private static final int EMPTY = 0; // 空位置
private static final int BLACK = 1; // 黑方
private static final int WHITE = 2; // 白方
private int[][] board = new int[ROWS][COLS]; // 棋盘状态
private int currentPlayer = BLACK; // 当前玩家,默认为黑方
private JLabel statusLabel; // 状态栏标签
public Gobang() {
setTitle("五子棋"); // 设置窗口标题
setSize(WIDTH, HEIGHT); // 设置窗口大小
setResizable(false); // 禁止调整窗口大小
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 关闭窗口时退出程序
JPanel boardPanel = new JPanel() {
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
drawBoard(g);
drawPieces(g);
}
};
boardPanel.setBackground(Color.WHITE); // 设置背景色
boardPanel.setPreferredSize(new Dimension(WIDTH, HEIGHT)); // 设置大小
boardPanel.addMouseListener(this); // 添加鼠标事件监听器
statusLabel = new JLabel("黑方先手");
statusLabel.setFont(new Font("宋体", Font.PLAIN, 16)); // 设置字体大小
statusLabel.setHorizontalAlignment(SwingConstants.CENTER); // 居中对齐
statusLabel.setPreferredSize(new Dimension(WIDTH, 30)); // 设置大小
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(boardPanel, BorderLayout.CENTER);
contentPane.add(statusLabel, BorderLayout.SOUTH);
}
// 绘制棋盘
private void drawBoard(Graphics g) {
g.setColor(Color.BLACK);
for (int i = 0; i < ROWS; i++) {
g.drawLine(MARGIN, MARGIN + i * CELL_SIZE, MARGIN + (COLS - 1) * CELL_SIZE, MARGIN + i * CELL_SIZE);
}
for (int i = 0; i < COLS; i++) {
g.drawLine(MARGIN + i * CELL_SIZE, MARGIN, MARGIN + i * CELL_SIZE, MARGIN + (ROWS - 1) * CELL_SIZE);
}
}
// 绘制棋子
private void drawPieces(Graphics g) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
int x = MARGIN + i * CELL_SIZE - PIECE_SIZE / 2;
int y = MARGIN + j * CELL_SIZE - PIECE_SIZE / 2;
if (board[i][j] == BLACK) {
g.setColor(Color.BLACK);
g.fillOval(x, y, PIECE_SIZE, PIECE_SIZE);
} else if (board[i][j] == WHITE) {
g.setColor(Color.WHITE);
g.fillOval(x, y, PIECE_SIZE, PIECE_SIZE);
g.setColor(Color.BLACK);
g.drawOval(x, y, PIECE_SIZE, PIECE_SIZE);
}
}
}
}
// 判断是否胜利
private boolean isWin(int row, int col) {
int count = 1; // 连续棋子数
int player = board[row][col];
// 水平方向
for (int i = col - 1; i >= 0; i--) {
if (board[row][i] == player) {
count++;
} else {
break;
}
}
for (int i = col + 1; i < COLS; i++) {
if (board[row][i] == player) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 垂直方向
count = 1;
for (int i = row - 1; i >= 0; i--) {
if (board[i][col] == player) {
count++;
} else {
break;
}
}
for (int i = row + 1; i < ROWS; i++) {
if (board[i][col] == player) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 左上到右下方向
count = 1;
for (int i = row - 1, j = col - 1; i >= 0 && j >= 0; i--, j--) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
for (int i = row + 1, j = col + 1; i < ROWS && j < COLS; i++, j++) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
// 右上到左下方向
count = 1;
for (int i = row - 1, j = col + 1; i >= 0 && j < COLS; i--, j++) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
for (int i = row + 1, j = col - 1; i < ROWS && j >= 0; i++, j--) {
if (board[i][j] == player) {
count++;
} else {
break;
}
}
if (count >= 5) {
return true;
}
return false;
}
// 判断是否平局
private boolean isDraw() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == EMPTY) {
return false;
}
}
}
return true;
}
// 切换玩家
private void switchPlayer() {
if (currentPlayer == BLACK) {
currentPlayer = WHITE;
statusLabel.setText("白方落子");
} else {
currentPlayer = BLACK;
statusLabel.setText("黑方落子");
}
}
@Override
public void actionPerformed(ActionEvent e) {
}
@Override
public void mouseClicked(MouseEvent e) {
int x = e.getX();
int y = e.getY();
int row = (y - MARGIN + CELL_SIZE / 2) / CELL_SIZE;
int col = (x - MARGIN + CELL_SIZE / 2) / CELL_SIZE;
if (row >= 0 && row < ROWS && col >= 0 && col < COLS && board[row][col] == EMPTY) {
board[row][col] = currentPlayer;
if (isWin(row, col)) {
JOptionPane.showMessageDialog(this, (currentPlayer == BLACK ? "黑方" : "白方") + "获胜!");
System.exit(0);
} else if (isDraw()) {
JOptionPane.showMessageDialog(this, "平局!");
System.exit(0);
} else {
switchPlayer();
repaint();
}
}
}
@Override
public void mousePressed(MouseEvent e) {
}
@Override
public void mouseReleased(MouseEvent e) {
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseExited(MouseEvent e) {
}
public static void main(String[] args) {
Gobang game = new Gobang();
game.setVisible(true);
}
}
```
该示例中,使用了 Java 的 Swing 库实现了一个简单的界面,棋盘和棋子的绘制通过 `paintComponent` 方法实现,鼠标事件监听器实现了用户与界面的交互。游戏逻辑包括判断胜利、平局以及切换玩家等。在 `main` 方法中创建游戏实例并显示窗口。
阅读全文
相关推荐
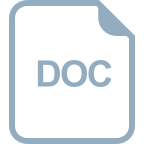
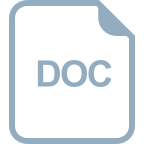
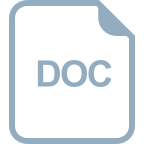

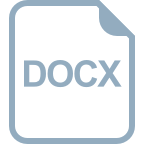
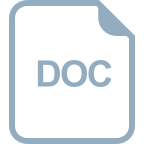
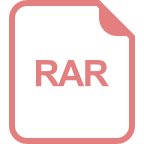
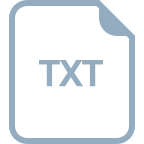



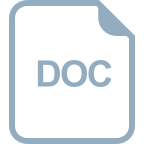
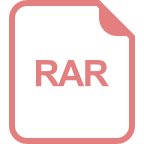
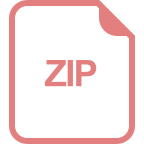
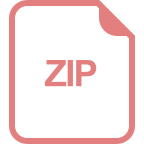
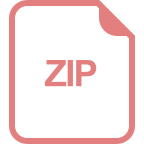
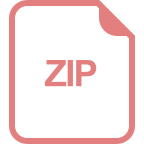