ssm用hadoop查看,上传,下载,删除文件前后端代码
时间: 2024-05-08 07:21:24 浏览: 107
1. 前端代码
上传文件:
```html
<form enctype="multipart/form-data" method="post" action="/file/upload">
<input type="file" name="file"/>
<input type="submit" value="上传">
</form>
```
下载文件:
```html
<a href="/file/download?fileName=文件名">下载</a>
```
删除文件:
```html
<a href="#" onclick="deleteFile('文件名')">删除</a>
<script>
function deleteFile(fileName) {
if (confirm("确定删除该文件吗?")) {
$.ajax({
url: "/file/delete",
type: "POST",
data: {
fileName: fileName
},
success: function (data) {
alert(data.msg);
window.location.reload();
},
error: function () {
alert("删除失败");
}
});
}
}
</script>
```
2. 后端代码
上传文件:
```java
@RequestMapping(value = "/upload", method = RequestMethod.POST)
@ResponseBody
public ResponseData upload(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return ResponseData.error("文件不能为空");
}
String fileName = file.getOriginalFilename();
File dest = new File(hdfsPath + fileName);
try {
file.transferTo(dest);
FileSystem fs = FileSystem.get(new URI(hdfsUri), new Configuration());
fs.copyFromLocalFile(new Path(hdfsPath + fileName), new Path(hdfsUri + hdfsPath));
fs.close();
dest.delete();
return ResponseData.success("上传成功");
} catch (IOException | URISyntaxException e) {
e.printStackTrace();
return ResponseData.error("上传失败");
}
}
```
下载文件:
```java
@RequestMapping(value = "/download", method = RequestMethod.GET)
public void download(HttpServletResponse response, String fileName) {
try {
FileSystem fs = FileSystem.get(new URI(hdfsUri), new Configuration());
InputStream in = fs.open(new Path(hdfsUri + hdfsPath + fileName));
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=" + fileName);
IOUtils.copy(in, response.getOutputStream());
in.close();
fs.close();
} catch (IOException | URISyntaxException e) {
e.printStackTrace();
}
}
```
删除文件:
```java
@RequestMapping(value = "/delete", method = RequestMethod.POST)
@ResponseBody
public ResponseData delete(String fileName) {
try {
FileSystem fs = FileSystem.get(new URI(hdfsUri), new Configuration());
fs.delete(new Path(hdfsUri + hdfsPath + fileName), false);
fs.close();
return ResponseData.success("删除成功");
} catch (IOException | URISyntaxException e) {
e.printStackTrace();
return ResponseData.error("删除失败");
}
}
```
阅读全文
相关推荐







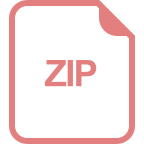
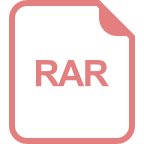
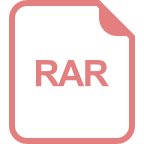