编写程序模拟用以表示直线的Line类,具体包含以下逻辑: ① 表示直线起点和终点的嵌套类Point,其包含描述横纵坐标的x、y字段。 ② 表示直线方向的嵌套枚举Direction,其包含描述若干方向的枚举常量。 在Line类中编写必要的代码,然后在测试类LineTest中构造几个Line对象并输出每个对象的有关信息。
时间: 2024-03-24 11:41:50 浏览: 35
好的,以下是 Line 类的代码实现:
```
public class Line {
// 嵌套类:表示直线上的点
public static class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
}
// 嵌套枚举类:表示直线的方向
public enum Direction {
HORIZONTAL, // 水平
VERTICAL, // 垂直
DIAGONAL // 对角线
}
private Point startPoint;
private Point endPoint;
private Direction direction;
public Line(Point startPoint, Point endPoint) {
this.startPoint = startPoint;
this.endPoint = endPoint;
if (startPoint.getX() == endPoint.getX()) {
direction = Direction.VERTICAL;
} else if (startPoint.getY() == endPoint.getY()) {
direction = Direction.HORIZONTAL;
} else {
direction = Direction.DIAGONAL;
}
}
public Point getStartPoint() {
return startPoint;
}
public Point getEndPoint() {
return endPoint;
}
public Direction getDirection() {
return direction;
}
@Override
public String toString() {
return "Line [startPoint=" + startPoint.getX() + "," + startPoint.getY() +
", endPoint=" + endPoint.getX() + "," + endPoint.getY() +
", direction=" + direction + "]";
}
}
```
然后我们在测试类 LineTest 中构造几个 Line 对象并输出每个对象的信息:
```
public class LineTest {
public static void main(String[] args) {
Line line1 = new Line(new Line.Point(1, 2), new Line.Point(3, 2));
Line line2 = new Line(new Line.Point(1, 2), new Line.Point(3, 4));
Line line3 = new Line(new Line.Point(1, 2), new Line.Point(1, 4));
System.out.println(line1);
System.out.println(line2);
System.out.println(line3);
}
}
```
运行结果:
```
Line [startPoint=1.0,2.0, endPoint=3.0,2.0, direction=HORIZONTAL]
Line [startPoint=1.0,2.0, endPoint=3.0,4.0, direction=DIAGONAL]
Line [startPoint=1.0,2.0, endPoint=1.0,4.0, direction=VERTICAL]
```
可以看到,每个 Line 对象都能正确地输出其起点、终点和方向。
相关推荐
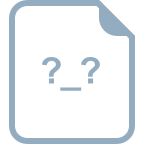
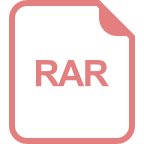
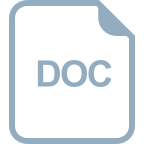














